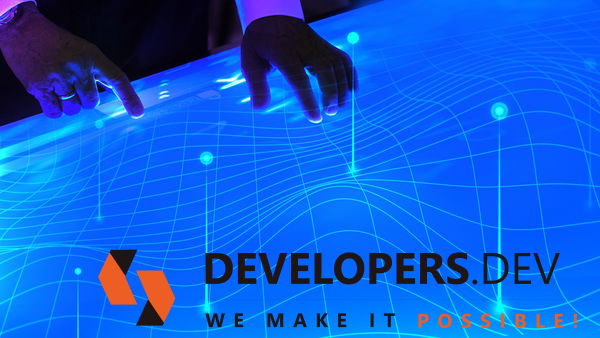
Effective memory management is not only essential to Java development but also a must. Although Java's garbage collector manages memory efficiently, developers are essential in optimizing this process for maximum benefit.
Achieving success requires in-depth knowledge of memory management methods and best practices, which may enhance applications' dependability and performance.
This article aims to offer an introduction to Java memory management, covering its architecture, workings, and how the Garbage Collector functions.
It also provides helpful advice for efficiently allocating memory resources. Regardless of your level of experience in developing Java apps, following this advice can help prevent frequent mistakes that plague most developers today and guarantee smooth operations of apps built using it.
Overview Of Java Memory Management
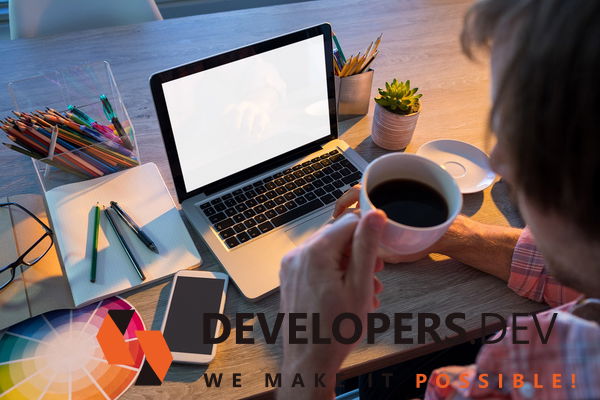
Memory is an indispensable and irreplaceable resource in computer languages; therefore, its management must be handled carefully without leakages and allocation/deallocation mistakes.
Memory allocation/deallocation in Java occurs under its Virtual Machine Management Architecture, with its Garbage Collector taking charge to ensure efficient handling without needing the programmer's intervention to allocate memory for them.
Memory management in Java can be an intricate balancing act. On one side lies the need to allocate enough memory.
Hence, your application runs efficiently while preventing excessive usage that leads to inefficiency or crashes. Achieving this balance requires knowledge, foresight, and an attentive eye particularly since other programming languages, such as C, allow direct access into the memory allocation, creating room for leakage issues.
Why Is It Important To Learn Java Memory Management?
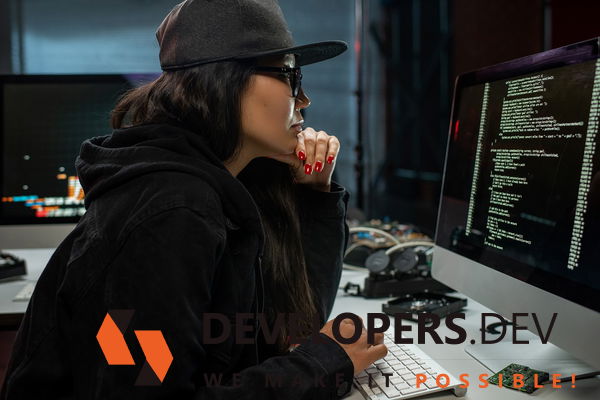
No one disputes that Java can manage memory independently without developers' programmatic intervention; garbage collection ensures unused spaces are cleaned up while memory may be released when no longer needed.
But what role should programmers play, and why is understanding Memory Management important for development teams?
Thanks to a garbage collector, programmers no longer need to fret over issues such as object destruction. Unfortunately, an automatic garbage pickup doesn't always guarantee everything; sometimes, we encounter JVM (Java Virtual Machine) managed objects if we do not fully grasp how memory works.
Garbage collection cannot handle everything automatically; therefore, having a firm understanding of memory management is vitally important to creating high-performance applications that do not crash or, should they crash unexpectedly, know how to repair themselves immediately.
Memory management refers to making space available for new object allocations by allocating new ones and deleting those no longer in use.
This section introduces object allocation, garbage collection, and memory management fundamentals within Oracle JRockit JVM. It provides basic memory management concepts and ideas.
Java Memory Management's Primary Concepts
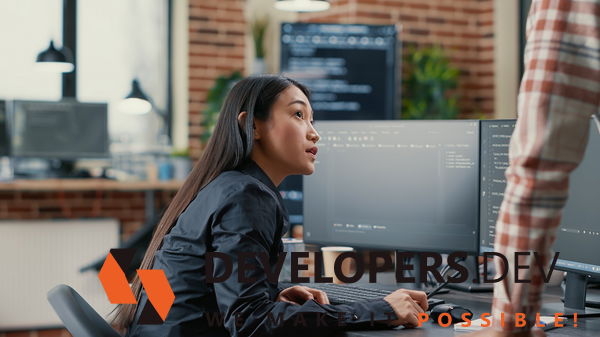
Main concepts of Java Memory Management involve Java Memory Structure and Garbage Collector Operation.
Java Memory Structure
JVM (Java Memory Management) defines various run-time data areas used during program execution. Threads within an application produce certain areas while JVM creates others - although its memory structures will only be deleted upon termination, unlike when threads instantiate data regions that will eventually disappear once their thread ceases executing.
Method Area
It is part of the heap memory that all threads share. It is created when a JVM starts up and stores class structures, superclass names, interface names, and constructor names within its method region.
JVM stores several types of data within this space:
- Name of an object.
- Names and components of Type superclass names.
- An exhaustive listing of fully qualified names of super interfaces.
Heap Area
Items stored within an object are kept within its respective heap area. At startup time, when Java Virtual Machine starts up, JVM creates a heap automatically; otherwise, its size can be fixed or variable as its users need.
When an instance of an object is created using new keyword syntax in JVM and stored in its associated heap, its reference remains on the stack; there's only one heap per JVM process active. As soon as its mound becomes fuller, it gets collected accordingly.
This statement creates a StringBuilder class object. The reference SB allocates to the stack while the object is allocated on the heap.
These sections comprise one heap: Young Generation, Survivor Space, and Old Generation/Permanent Generation Cache Caches can all be found.
Stack Area
As soon as a thread starts running, its stack area is created, either fixed or variable in size, depending on which thread started it.
It is used to store data and incomplete results. Each thread receives its own allocated stack memory, which contains specific items that may be mentioned. Additionally, it stores values themselves rather than references to heap objects; its visibility depends on its "scope."
Native Method Stack On Java
Unfortunately, Java does not support native method stacks or C stacks; when creating threads, they receive their memory allocation, which could be fixed or dynamic.
Register Of Program Counter (PC)
Every JVM thread that executes the task for any method connects to a program counter register (PC). While native methods have unknowable PC values, non-native ones contain addresses of accessible JVM instruction blocks and native pointer or return address storage capabilities, depending on platform availability.
How A Garbage Collector Works
Java programs use memory in various ways as they run, with the heap serving as the repository of items stored in memory that participate in trash collection - sometimes known as a garbage collector heap - taking up as little room as possible due to all this activity, finding and collecting anything out-of-reach falls to its purveyance.
What Capabilities Is Java Garbage Collector Equipped With?
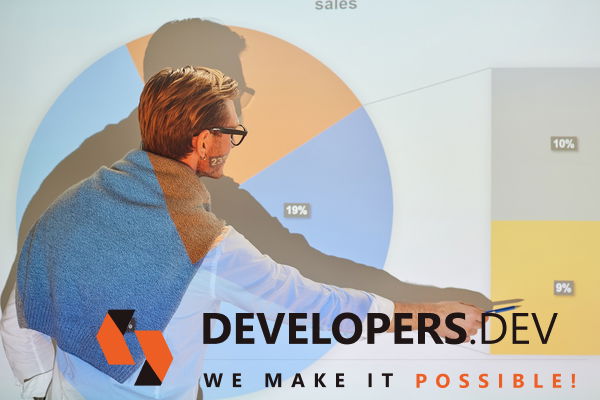
The JVM oversees its garbage collector system. It determines when trash collection should occur and can also be instructed by us.
However, no guarantee can be given for its implementation. When memory becomes scarcer than expected, garbage collection begins immediately. In contrast, requests from Java programs for garbage collectors are often met swiftly.
However, not every demand can be fulfilled immediately.
Read More: Transform Your Business with Java App Development
Garbage Collection Process
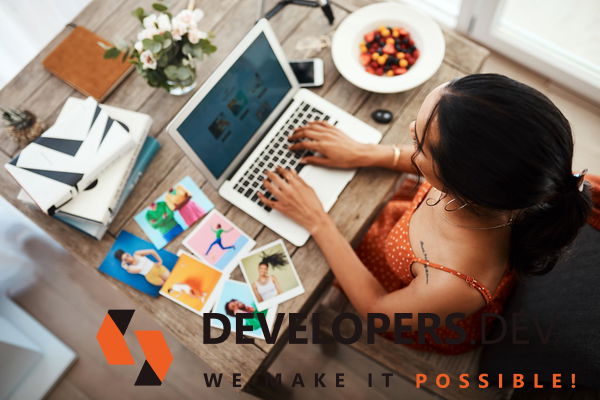
As was discussed previously, depending on the reference type used between variables on the stack and objects from the heap, particular objects become eligible for garbage collection at specific points in time.
As an example, all objects highlighted with red are eligible to be collected by the garbage collector. You might notice an object on the heap with solid references to another (perhaps it contains references for its items or two referenced type fields).
Still, once its stack reference has gone away, it becomes garbage. Before we dive deeper, here's some further detail for understanding purposes:
- Java is responsible for initiating this process automatically; when and if to initiate it depends entirely on Java itself.
- As it can be expensive, running the garbage collector requires pausing all threads within your application.
- This process goes far beyond simply collecting garbage and freeing up memory.
Garbage Collection Types
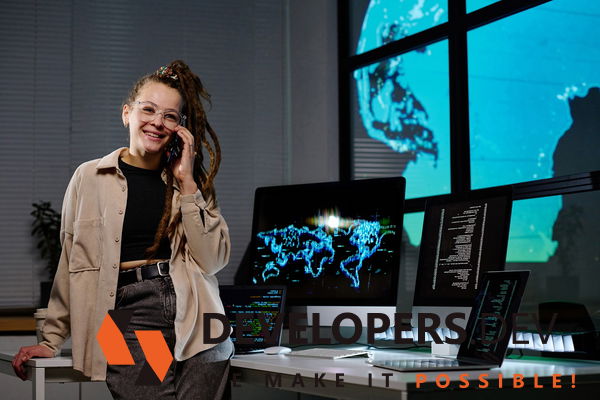
Here are five types of garbage collection services:
- Serial GC: Serial GC utilizes the mark-and-sweeps technique (minor/major GC) to cater to young and elderly generations, respectively.
- Parallel Garbage Collection: As with serial garbage collection, parallel garbage collection involves creating N (the number of CPU cores in your system) threads for new-generation trash pickup.
- Parallel Old GC: The only distinction between parallel old GC and old GC is that old GC uses multiple threads for both generations of its production process.
- Concurrent Mark Sweep (CMS) Collector: Concurrent Mark Sweep Collector (CMS) collects waste for seniors by setting the Parallel CMS Threads.
- G1 Garbage Collector: Java 7 saw its introduction, intending to take over from CMS collectors. As its name implies, this garbage collector operates concurrently and parallel with CMS collection; no area exists between young and senior generations, and piles are divided equally across size scales. The first regions for collection will contain minimal live data.
Best Practices In Memory Management
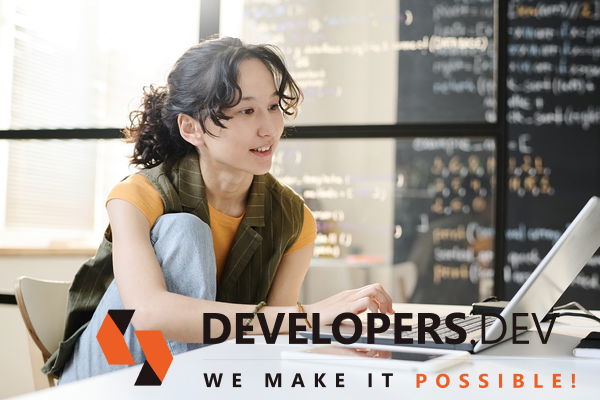
Effective memory management is a critical element of Java application development. Although Java's Garbage Collector (GC) provides a solid framework for handling memory usage, developers still play an essential part in ensuring all available memory is utilized effectively.
This section discusses critical practices that should be observed to achieve efficient memory use within applications developed using the language.
- Effective Object Generation And Management Techniques: Java objects must be created carefully; every object consumes heap space, so excessive creation could result in memory bloat, compromising performance.
- Judicious Object Creation: One key strategy is creating objects only when necessary. Using primitives instead of wrapper classes where possible may save memory, while singleton and factory patterns may reduce the number of instances created.
- Utilizing Object Pools: Object pools can provide invaluable assistance in environments where objects are often created and destroyed. Managing instances that can be reused without increasing object creation costs or garbage collection overhead.
- Utilizing Data Structures Effectively: The selection of an optimal data structure is also vital. Using an ArrayList for frequent additions and deletions within its body would be inefficient compared to a linked list. Understanding when weak references or soft references might help in memory-sensitive situations also contributes to success.
- Preventing Memory Leaks: Java memory leaks can often be subtle and hard to spot, especially since users often do not see their symptoms. They occur when objects no longer needed are referenced, preventing the garbage collector (GC) from freeing up space allocated by these objects.
- Recognition And Fixing Of Memory Leaks: Profiling applications regularly is vital to identifying memory leaks and leakage points, with tools like VisualVM, Eclipse Memory Analyzer, and heap dumps analyzers providing invaluable aid. Once identified, it's important to dive deep into code analysis to discover why objects aren't being released as expected and correct this reference problem as quickly as possible.
Want More Information About Our Services? Talk to Our Consultants!
Conclusion
Effective memory management is integral to Java development services, impacting performance and reliability.
This article examines the intricate details of Java's memory structure, the workings of its Garbage Collector, and strategies for efficient memory administration. By understanding heap and stack sizes and tuning garbage collection to best practices in object management and leak prevention techniques, developers can significantly increase the success of their apps.