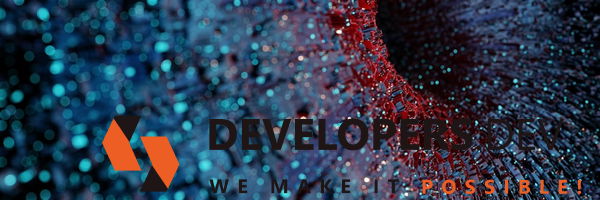
Rails is another name for Ruby on Rails. It serves as a popular framework for web programs written in the Ruby programming language.
Full-stack apps can be easily made using the web application framework Rails. Many built-in functionality can be used by developers thanks to Rails. We can handle routing, use a template engine, and link to databases, for instance.
The Model-View-Controller (MVC) architecture paradigm is one of the main components of Rails. This pattern separates an application into three separate sections:
- The model manages application data.
- The view handles the presentation of data (i.e., the UI).
- The controller, which controls the flow.
Developers find it simpler to organize and maintain their source code. Developers may divide concerns between user interface (UI) and business efficiency logic thanks to Rails MVC architectural design.
The controller manages how the User interacts with the software, whereas the model is the logic and the view is the presentation layer. One of the most popular web frameworks is MVC. However, other coding standards serve as the basis for Ruby on Rails.
Convention over Configuration (CoC) and Do not Repeat Yourself (DRY) are two software engineering concepts that Rails encourages developers to follow.
Rails developers are responsible for designing and building the structure of web apps using the Ruby on Rails Framework.
It includes setting up URL routes and creating database schemas. Also, they write the code to connect the app to the database and manage user interactions. Rails developers collaborate closely with other developers and the graphics team to make sure the final product is what the client wants.
The developers may be responsible for testing and debugging an application to make sure it functions as intended.
Additionally, over time, Rails developers must maintain the apps security and updates. It includes enhancing the apps functionality, eliminating bugs, and making sure users are protected.
They must be knowledgeable with the most recent tools and technologies that will enable them to integrate features like authentication and authorisation, among others. The Ruby on Rails Framework and the world of web development as a whole are seeing trends and improvements that Rails developers need to be aware of.
They must be well-versed in web design principles including the MVC (model-view-controller pattern), RESTful Architecture, and Agile methodologies.
The convention over configuration tenet is the foundation of the Ruby on Rails framework. Because they do not have to repeat the code they developed, developers believe RoR to be simple to use.
Developers make choices while creating apps that may appear crucial at the moment but end up being harmful. When you select RoR for your development framework, it is not clear that everything will run smoothly and the project will be successful.
It is important to pay attention and take care of the development process to ensure that it runs smoothly. If you are concerned about the performance of your application, hire Ruby on Rails programmer to look into it before it affects your business.
RoR performance issues can sometimes be as simple or complex as you like. You should contact a developer that works on Ruby on Rails. App owners can handle things like web hosting - they just need to change the web host.
Ruby On Rails Performance Tips
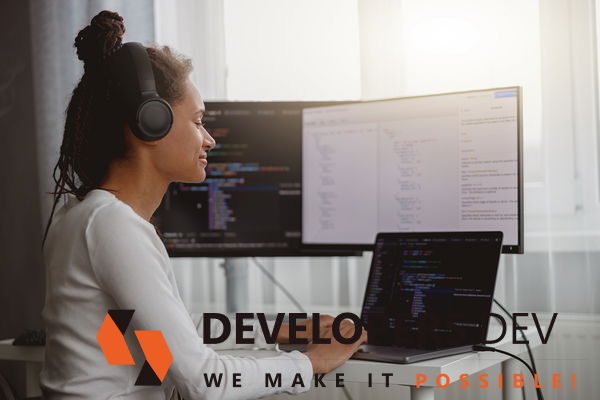
The Website Host:
You should always check the host website when evaluating the performance of a Ruby on Rails app. You might have used a variety of tools and resources to test the RoR application.
You may have tested the app in a development environment, and it could have worked well. After deployment, users start complaining that the pages are loading slower than normal. Your RoR apps performance can also be affected by the web hosting service.
Consider changing your app hosting service provider.
Use the Content Delivery Network service. This is a network that has a minimal amount of hops and a 100% guaranteed data packet delivery.
Google Cloud Platform or Amazon Web Services (AWS), which offer reliable and scalable options, can assist you in deploying RoR. Heroku is another option that you may consider, as it allows for faster app launches. Digital Ocean is a good option if you need a high level of control over your app.
It provides bundles called droplets which are useful for running production apps.
Check The N+1 Query:
An N+1 query could be one of the causes of slow performance in your app. This query requires a solution. The bullet is a Ruby gem that can be used to improve app performance.
When an app becomes larger, it will try to run multiple queries in order for a positive result. This is more efficient than collecting related data with one query. The N+1 problem is difficult to detect because the performance problems are only apparent after moving to a larger database.
Bullet gem can be used to identify N+1 queries that could be causing the slow performance of your app. can hire a Ruby on Rails developer who will check to see if N+1 queries are causing the slow performance of your app.
Include Indexes In The Database:
Traffic problems can affect the performance of an application or website. Indexes in the database can help you fix traffic problems.
It would be very slow to run some queries without indexes in columns. The engines of the database might have to manually check each Record to find a match without indexes. When you add indexes to the database, this process is done quickly so that you can focus on choosing the best solution.
If you are having problems, ask Ruby on Rails specialists for help.
Use Gems And Plugins:
If you want to increase your applications speed, you should use gems and plugins. It is important to familiarize yourself with gems and plugins for RoR applications.
Rails plugins are available in the growing RoR development community. They can save you a lot of time. You should do some research before using any plugins. Plugins are both solutions and problems.
Many plugins and gems can be used to optimize the RoR code.
Turn On Caching:
You can turn on caching if youve tried gems and plugins but havent succeeded. Rails caching can be turned on to cache assets, pages, fragments, and SQL requests.
You can choose what you want for your particular case. If you cache data with active records, you can store user requests directly in the devices memory without having to connect it to a DMS.
Server Optimization:
The performance of an application can be affected by many factors, including memory capacity, web hosting, and the quality of coding.
You can check server capabilities if you notice a decrease in performance due to increased web app users. You might want to increase RAM to get your app running faster. You may also want to upgrade your computer.
Load balancing can help improve performance. Apps that are used by high traffic can process thousands of requests per second.
When multiple requests are processed simultaneously, it isnt easy to get a response instantly. Load balancing can help you route requests to servers appropriately. App performance will improve, and youll get a faster app.
You can also keep your code neat. To accomplish this, the developer must create the apps logic in a timely manner and adhere to clearly defined code structures.
Ensure the functions are named according to standard principles and follow a strict naming convention.
Optimize Your Ruby Code
Rails applications are essentially ruby coder that must be executed.
Be sure that your code is efficient when viewed from the Ruby perspective. Ask yourself if you need to refactor your code, considering performance and algorithmic efficiency. Although profiling tools can be very useful in identifying slow codes, here are a few general considerations that you may not have considered before:
- Use the built-in methods and classes when available instead of creating your own.
- When you want to process and parse all text, use Regular Expressions instead of expensive loops.
- If you need to process XML documents, use Libxml instead of the slower REXML.
- You may sometimes want to sacrifice a little elegance or abstraction in favor of speed. It can be expensive to define_method or yield;
- If possible, remove slow loops. You can avoid loops in some cases by restructuring your code.
- Hash tables are costly data structures. If you want to retrieve the value of a key several times, consider storing it in a variable. In general, you should store any data structure that is frequently accessed in a local, instance, or class variable.
Caching Is Good For You
Caching can speed up your application. Particularly:
- You can cache your models using the gem cached_model or the plugin act_as_cached.
- Use MemCacheStore for Sessions containers.
- Cache your pages through fragment cache (take a look at the extended_fragment_cache).
Use Your Database As Far As The Law Allows:
Dont be scared to use the cool features that your database provides, even if Rails doesnt directly support them.
This will allow you to bypass ActiveRecord. You can define stored functions and procedures, knowing you can access them directly through the database using driver calls instead of ActiveRecords high-level methods.
This can dramatically improve the performance and reliability of Rails applications that are data-bound.
The 10 Best Finders, But Beware:
Finders are easy to use and dont require any SQL knowledge. The nice high-level abstraction comes at a cost. Use these simple rules:
- Only retrieve the data you require. Selecting data that you dont need can waste a lot of time. Use the appropriate options when using the finders to only select the required fields (: select). If you only require a subset of numbered records, specify the limit with the limit or offset options.
- Avoid dynamic finders such as MyModel.find_by. While using something like User.find_by_username is very readable and easy, it also can cost you a lot. ActiveRecord generates these methods dynamically within method_missing, which can be very slow. The mapping between the model attribute and the method (in our example, username) is achieved by a select query that is created before the data is sent to the database. It is more efficient to use MyModel.find_by_sql or MyModel.find directly.
- Use MyModel.find_by_sql to optimize your SQL queries. Even if the SQL statement is the same in the end, find_by_sql will be more efficient. (No need to construct the SQL string using the options passed to the method.) You can use Find if youre building a cross-platform plugin, but make sure that your SQL queries work on all Rails databases. Find is generally more readable and produces better code. Before you start filling your application with find by sql, do some profiling to identify slow queries that may need to manually be optimized and customized.
Group Operations Within A Transaction
Active record wraps up the creation or updating of a record into a single transaction. Multiple inserts generate multiple transactions (one per insert).
Multiple inserts can be grouped into one transaction to speed up the process.
Instead of:
- Filters are expensive. Dont abuse them. Dont use too many instance variables if they arent actually needed by your views.
- In your view templates, dont use too many helpers. Each time you use a form helper, you add an extra step. You may not need someone to create the HTML code for you. You may even make your designer happy if he doesnt know Ruby!
- Logging is expensive and at an inappropriate level (e.g., logging all information). Logging can be expensive, and the wrong level (e.g., Logger: DEBUG can cause your production application to be crippled.
- Patch the GC. This is not a coding problem, but its strongly recommended that you patch Rubys Garbage Collection. It will significantly improve the performance of your Ruby and Rails application.
- Final note: While we do not advocate premature optimization (but also dont go overboard), if possible, try to work on your code using these principles. It is possible to make last-minute changes, but they are less desirable than "performance-aware" coding.
Ruby On Rails Advantages

Note the following attributes and advantages of Rails to get a better understanding of why you may want to use it:
Flexibility
Rails, like RoRs base language, is easy to use and flexible. RoRs flexibility is a result of its extensive use of meta-programming.
Metaprogramming in Ruby is a technique that allows programs to behave as data. Ruby developers are able to write code that can be executed during runtime in order to resolve issues without the need for recompilation.
For example, if a developer forgets to define a method before runtime for whatever reason, the Rails Framework will create a method dynamically using the database.
This effectively eliminates any errors. The term monkey patch describes a common occurrence as part of RoR development metaprogramming.
A program will extend or modify a class in order to accommodate new data.
Speed Up
Rails internal structure is based on a variety of design principles and software principles that help businesses to develop faster.
Ruby on Rails comes with everything you need. RubyGems is the official package manager for RoR. Developers can use it to access a wide range of third-party components.
The layered structure is another huge advantage. In addition to Railss MVC, RoR provides three standard environments - development, testing, and production - optimizing the software life cycle.
Rails also produce scaffolding. This is a computer programming code generation method for database access. By using scaffolding, developers can create models, views, and controllers of a new resource in a single operation.
Here is an example of CoC being used. Software development places a high priority on delivering software quickly. Clients want to see regular updates with new features.
A rapid time-to-market (TTM), in addition to lowering development costs and better adapting to changing markets, also provides quick feedback from users.
Cost-Effectiveness
Ruby on Rails, first and foremost, is an open-source project. This means that you can avoid licensing fees. RoRs modularity, which is provided by gems, mixes, blocks, and other useful features, allows developers to save time during development.
Software development is a perfect example of the classic business maxim time equals money. Ruby on Rails is said to accelerate development by up to 40%. Ruby on Rails can save you money.
Testability
Ruby on Rails makes it easy to test your software. RoR comes with built-in tests. Rails provide automated tests that you can extend to your liking.
The framework will produce a skeleton of test code while you are busy creating controllers and models. Similar Rails features include harnesses and fixtures. These are supporting codes for creating and running test cases.
The rake tool allows developers to execute all of their automated tests simultaneously.
Ruby On Rails Has Its Drawbacks
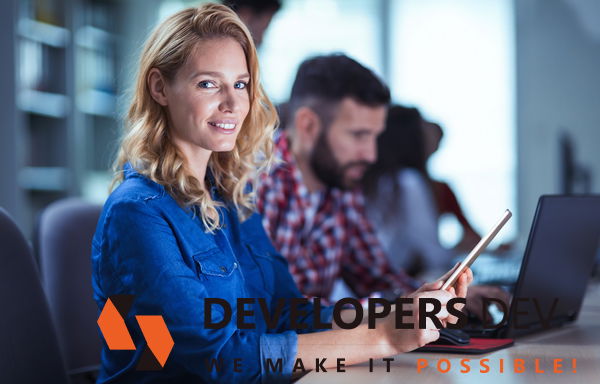
Like all technologies, Ruby on Rails has some disadvantages. We will now explore the main disadvantages of Ruby on Rails.
Runtime Speed
Ruby on Railss runtime speed is one of its biggest disadvantages. Ruby code is slower than other technologies like Node.js or Golang.
This can be a major drawback when working on projects requiring high performance and low latencies. This can result in longer loading times for end users, which negatively impacts their UX.
Lack Of Flexibility
Ruby on Rails lack of flexibility is another drawback. ROR is great for standard web apps, but it can be difficult to customize and add unique functionality to them.
The hard dependency between models and components is the reason for this. This hard dependency makes it very difficult to add new features and make changes without affecting the functionality of the entire application.
Boot Speed
Ruby on Rails boot time is another drawback. It takes a while to start due to the large number of files and gem dependencies.
This can affect developer performance. Slow boot speeds can cause delays in development and affect the developers productivity.
Documentation
Finding good documentation on Ruby on Rails is not always easy. This is especially true for gems that are less popular and libraries that heavily rely on mixins.
It can be difficult for developers, and even lead to delays, to learn how to use specific features.
Multithreading
Ruby on Rails is multithreaded, but certain IO libraries are not because they hold the global interpreters lock.
If youre not careful, you could end up with performance problems if your request is queued behind active requests. This can cause delays in application performance and have a negative impact on the Users experience.
Use Rails Or Not?
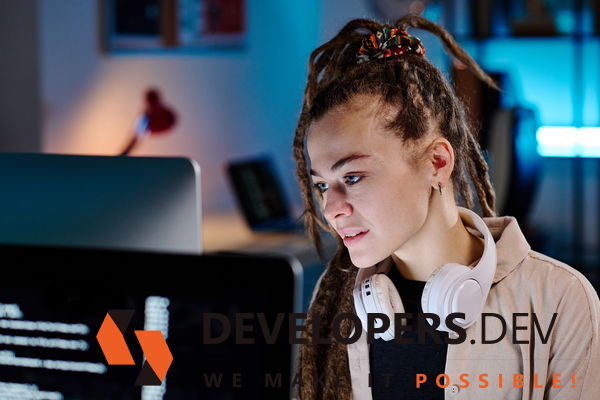
Rails have some limitations. Here are a few general scenarios in which Rails is useful for development.
Use Rails for:
- You can use this tool when youre building web applications that require many database interactions.
- You can use its conventions to speed up the development process.
- Suppose you are looking for a framework that has a large, active community. This can make it much easier to find resources and help.
- You can use it to express yourself or for its flexibility and readability.
Rails isnt a good solution in situations such as:
- If you want to create a low-latency, high-performance application such as a trading platform or high-end gaming system that handles millions of transactions per day.
- If you are building an application that requires a large amount of computing power (such as machine learning or scientific simulation).
- When developing an application that requires a large amount of concurrency and parallelism.
The framework chosen should always be determined by the requirements of the project as well as the experience of the team.
Conclusion
Ruby on Rails has many features that make it a powerful framework for web development. Ruby is a flexible language that promotes true object-oriented programming and aims to make programming enjoyable for developers.
Ruby on Rails is a natural extension of this goal. Rails is credited with helping many companies achieve global recognition, thanks to its speed and flexibility. Ruby on Rails is a crucial factor in reaping the benefits Rails has to offer.
You can do these things if you notice that your RoR apps performance is deteriorating in certain circumstances. It is important to test the app, but you may find that it behaves differently when its deployed in real life.
In the controlled test environment, there may not be many requests sent at the same time, but once you deploy the application, the traffic increases, which can slow it down. You may need to hire a Ruby on Rails experts developer who has extensive knowledge of RoR Development if youre experiencing performance problems with your Ruby on Rails application.
Keep in mind that performance issues can have a negative impact on your business. Todays audiences want a faster experience and will abandon web apps or pages that load slowly. This could lead to a loss of revenue.