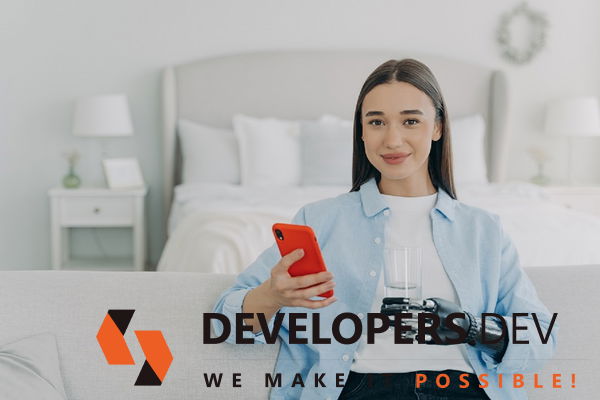
In the contemporary digital landscape, mobile applications have become indispensable for communication, commerce, and entertainment.
Central to these applications are APIs (Application Programming Interfaces), which serve as the conduit between a mobile app's user interface and its backend systems. APIs facilitate seamless data exchange, user authentication, and integration with services like payment gateways and location tracking.
Whether it's logging into a banking app or retrieving real-time product information, APIs ensure these interactions are conducted securely and efficiently.
They also promote scalability and maintainability by encouraging modular, reusable code structures.
As mobile applications continue to permeate various industries, a comprehensive understanding of API development becomes crucial.
This guide delves into fundamental API concepts, architectural considerations, development steps, best practices, and common pitfalls to avoid, equipping developers and businesses with the knowledge to build an API for a mobile app effectively.
Deciphering APIs and Their Role in Mobile Applications
An Application Programming Interface (API) comprises a set of defined rules and protocols that enable different software applications to communicate.
In mobile app development, APIs allow the frontend, the part users interact with, to communicate with backend systems like servers and databases.
The client-server model underpins this interaction. Here, the mobile app (client) sends requests to the server, which processes them and returns appropriate responses.
This architecture ensures efficient data management, as the server handles intensive tasks, keeping the mobile app responsive.
APIs are vital for integrating various functionalities into mobile apps. For instance, integrating Google Maps API enables location-based services, while payment gateway APIs facilitate secure transactions.
Social login APIs from platforms like Facebook or Google streamline user authentication processes. These integrations not only enhance app capabilities but also improve user experience.
Moreover, APIs support the scalability and maintainability of mobile applications. By decoupling the frontend and backend, developers can update or modify one component without impacting the other.
This modularity is essential for continuous development and deployment, allowing for rapid updates and feature additions.
In essence, APIs are the backbone of modern mobile applications, facilitating seamless communication between software components, integrating essential services, and supporting scalable and maintainable architectures.
Navigating API Types for Mobile Applications
Choosing the right API type is pivotal for a mobile application's performance and scalability. The primary API types utilized in mobile apps include RESTful APIs, GraphQL, SOAP, and WebSockets.
-
RESTful APIs: These are the most prevalent in mobile app development.
They employ standard HTTP methods and are stateless, meaning each client request contains all necessary information.
RESTful APIs are lauded for their simplicity and scalability, making them ideal to build API for mobile app.
- GraphQL: This query language allows clients to request specific data, minimizing data transfer over networks, a boon for mobile apps with limited bandwidth. GraphQL also enables clients to aggregate data from multiple sources in a single request, simplifying client-side data management.
- SOAP (Simple Object Access Protocol): A protocol offering higher security levels, often used in enterprise applications. However, its complexity and heavier payloads make it less suitable for mobile apps, which demand lightweight and efficient communication protocols.
- WebSockets: These facilitate real-time, two-way communication between client and server, essential for applications requiring instant data updates, like chat apps or live sports scores. WebSockets maintain an open connection, allowing continuous data exchange without repeated HTTP requests.
Each API type offers distinct advantages and is selected based on the mobile application's specific requirements.
Understanding these types enables developers to design APIs that align with their app's functionality and performance needs.
Crafting API Architecture for Mobile Applications
Designing an effective API architecture is crucial for a mobile application's success. It requires meticulous planning to ensure scalability, security, and maintainability.
- Defining Functionalities: Start by outlining the app's core features, such as user authentication, data retrieval, or payment processing. This step aids in identifying necessary endpoints and data models.
- Endpoint Structuring: Design clear and consistent endpoints adhering to RESTful conventions. For example, use /api/v1/users for user-related operations. Consistent structuring simplifies integration and maintenance.
- Data Formatting: Opt for lightweight data formats like JSON to ensure efficient data exchange between client and server. JSON's wide support and ease of parsing make it suitable for mobile applications.
- Authentication Mechanisms: Implement robust authentication protocols, such as OAuth 2.0, to secure user data and prevent unauthorized access. Token-based mechanisms like JWT (JSON Web Tokens) effectively maintain secure sessions.
- Scalability Planning: Design the API to handle increased loads by incorporating caching strategies and load balancing. Implementing rate limiting and monitoring tools helps manage traffic and ensure consistent performance.
By thoughtfully planning the API architecture, developers can establish a robust foundation for their mobile applications, ensuring seamless functionality and a positive user experience.
Step-by-Step Guide to Developing an API for a Mobile App
Developing a robust API for a mobile app involves several critical steps, each contributing to the application's overall functionality and performance.
- Selecting the Technology Stack: Choose backend technologies like Node.js, Python (with frameworks like Django or Flask), or Java, and databases such as PostgreSQL or MongoDB, based on the app's requirements. Consider factors like scalability, community support, and ease of integration.
- Setting Up the Development Environment: Configure the development environment with the necessary tools and frameworks. Establish version control using Git to manage code changes and facilitate collaboration.
- Creating and Structuring API Endpoints: Define endpoints corresponding to the app's functionalities, ensuring they follow RESTful conventions for consistency. For instance, use GET /api/v1/users to retrieve user data.
- Implementing Authentication and Authorization: Secure the API using authentication protocols like OAuth 2.0 and implement role-based access controls. Token-based authentication mechanisms like JWT effectively maintain secure sessions.
- Integrating with Mobile App Frontends: Utilize libraries like Retrofit for Android or Alamofire for iOS to facilitate communication between the app and the API. These libraries simplify making network requests and handling responses.
- Testing the API: Employ tools like Postman or Swagger to test API endpoints, ensuring they function correctly and handle errors gracefully. Conduct unit and integration testing to validate the API's performance under various scenarios.
- Deploying and Monitoring: Deploy the API to a reliable hosting service, such as AWS or Heroku, and set up monitoring tools to track performance and uptime. Implement logging mechanisms to capture and analyze errors.
- Documenting the API: Create comprehensive documentation detailing endpoints, request/response formats, and error codes to assist developers in integration. Tools like Swagger and Redoc can help generate interactive documentation.
By following these steps, developers can build API for mobile app that is secure, scalable, and efficient, serving as a solid foundation for their mobile applications.
Best Practices for API Development in Mobile Applications
Developing robust APIs for mobile applications necessitates adherence to best practices that ensure scalability, security, and maintainability.
- Versioning: Implement version control from the outset, such as including /v1/ in API endpoints. Semantic versioning (v1.0.0, v1.1.0, etc.) provides clarity on the nature of changes, distinguishing between major updates, minor enhancements, and patches.
- Securing Endpoints: Employ authentication mechanisms like OAuth 2.0 and token-based systems (e.g., JWT) to ensure only authorized users can access the API. Additionally, validate all inputs to prevent injection attacks and enforce HTTPS to protect data in transit.
- Caching Strategies: Implement caching mechanisms, such as HTTP caching headers or utilizing tools like Redis, to reduce server load and improve response times. This is especially beneficial for endpoints serving data that doesn't change frequently.
- Rate Limiting and Monitoring: Control the number of requests a client can make within a specific timeframe to prevent abuse and ensure consistent performance. Monitoring tools can help track usage patterns and detect anomalies.
- Error Handling Conventions: Provide clear and consistent error messages, along with appropriate HTTP status codes, to aid in debugging and enhance the API's usability.
Adhering to these best practices in API development for mobile apps ensures the creation of reliable and efficient APIs.
Common Mistakes to Avoid During API Development
Even experienced developers can encounter pitfalls when designing APIs for mobile applications. Avoiding these common mistakes can significantly enhance the functionality, performance, and user experience of your app.
- Neglecting API Versioning: Failing to version APIs can create compatibility issues when changes are introduced. Always start with versioning in the URI (e.g., /api/v1/) to ensure smooth transitions for existing clients.
- Weak Security Measures: Skipping proper authentication and authorization can expose sensitive data to unauthorized access. Implement secure protocols like OAuth 2.0 and enforce HTTPS for all communications.
- Poor Error Handling: Returning vague or unstructured error messages makes debugging difficult and frustrates end users. Use standardized HTTP status codes and provide informative error messages.
- Inconsistent Endpoint Naming: Using unclear or inconsistent endpoint structures can confuse frontend developers. Stick to RESTful naming conventions and maintain consistency across the API.
- Over-fetching or Under-fetching Data: Not optimizing data payloads can either lead to unnecessary data being transmitted or force additional API calls. Tools like GraphQL can help fine-tune data retrieval.
- Ignoring Performance Optimization: Not implementing caching or compression strategies can slow down the app. Utilize GZIP compression and appropriate caching headers to enhance speed.
Being mindful of these issues early in the development process can prevent costly revisions and ensure a smoother user experience.
Emerging Trends in API Development for Mobile Apps
As mobile technologies evolve, API development is also adapting to meet the demands of performance, scalability, and user expectations.
- Serverless Architectures: Platforms like AWS Lambda allow developers to run backend logic without managing servers. This enables scalable and cost-effective API deployment.
- API-First Development: More teams are adopting an API-first approach, where APIs are designed before the frontend and backend development begins. This ensures better alignment across development teams and promotes reusable code.
- Event-Driven APIs: Using event-driven patterns (e.g., Webhooks or Kafka) allows APIs to respond to events in real-time, which is ideal for apps requiring instant updates or notifications.
- Increased Use of GraphQL: GraphQL is becoming popular due to its ability to let clients request exactly what they need-no more, no less. This reduces over-fetching and under-fetching, which is especially helpful in mobile environments.
- Enhanced API Security: With increased cyber threats, developers are integrating advanced security practices like OAuth 2.1, Zero Trust architecture, and encrypted tokens.
- API Observability Tools: More projects are adopting observability platforms like Datadog, New Relic, or Prometheus to monitor API health, traffic patterns, and errors in
Case Studies: Real-World Examples of Successful API Implementation
To understand the practical applications of API for mobile app development, let's look at a few real-world examples of companies that have successfully built and scaled mobile apps with robust API infrastructures.
- Spotify: Spotify utilizes RESTful APIs to manage music libraries, user playlists, and streaming history. These APIs allow seamless integration between mobile and desktop apps, enabling users to switch devices without interruptions. Their API for mobile app strategy focuses heavily on real-time data delivery and performance optimization.
- Uber: Uber's mobile app uses a combination of RESTful and WebSocket APIs to provide real-time ride updates, location tracking, and fare calculations. Its API for mobile app functionality is critical in delivering a frictionless experience for both drivers and riders, especially under fluctuating demand conditions.
- Instagram: The Instagram API facilitates content sharing, social interactions, and third-party integrations. Instagram's API for mobile app structure supports high traffic and handles media uploads, comments, likes, and messaging, all while maintaining high performance and minimal latency.
These case studies highlight the impact of well-designed APIs in enabling responsive, feature-rich mobile experiences.
When you build API for mobile app, it's essential to ensure high availability, fast response times, and tight integration with core services, as demonstrated by these tech giants.
Tools and Frameworks to Simplify API Development
Using the right tools and frameworks can significantly streamline the process to build API for mobile app. Here's a look at some popular options for backend development, API testing, and mobile integration:
-
Backend Frameworks:
- Node.js with Express: Lightweight and efficient, ideal for building RESTful APIs.
- Django (Python): Offers built-in features like authentication, making it a secure choice.
- Spring Boot (Java): Great for enterprise-scale applications with advanced features.
-
API Testing Tools:
- Postman: Widely used for developing, testing, and documenting REST APIs.
- Swagger: Allows you to design and document APIs interactively, aiding collaboration.
-
Mobile Integration Libraries:
- Retrofit (Android) and Alamofire (iOS): Help connect the mobile frontend with backend APIs efficiently.
-
API Management Platforms:
- Apigee, Kong, and AWS API Gateway offer features like rate limiting, analytics, and version control to manage large-scale API for mobile app deployments.
These tools not only simplify the development and testing process but also ensure that your APIs are scalable, maintainable, and easy to integrate across different mobile platforms.
Leveraging these technologies can help reduce development time and ensure consistency when you build API for mobile app.
XI. Conclusion: Building Future-Proof APIs for Mobile Applications
As mobile apps continue to evolve, the importance of APIs will only grow. A well-designed and secure API for a mobile app serves as the backbone of functionality, user experience, and scalability.
From choosing the right architecture and technology stack to implementing security best practices and staying updated with emerging trends, every decision made during the API development process impacts the overall success of your app.
When you build an API for a mobile app, your focus should go beyond just getting endpoints to work; it's about creating a robust communication layer that is secure, maintainable, and scalable.
With technologies like GraphQL, serverless architecture, and advanced monitoring tools becoming more accessible, there's never been a better time to adopt best practices in API development.
Whether you're a startup or an enterprise, understanding the nuances of API development empowers your team to deliver responsive, high-performance mobile apps that users love.
Frequently Asked Questions (FAQs):
How long does it take to develop a custom API for a mobile app?
The time required to develop an API for a mobile app depends on the complexity of the app features, the number of endpoints, and the security or integration requirements.
A basic API with user authentication and CRUD operations may take 2-4 weeks, while a more advanced API with payment gateways, third-party integrations, and performance optimization can take 6-12 weeks or more.
Can APIs be reused across multiple mobile applications?
Yes, APIs can be reused across multiple apps or platforms, especially if they are built with scalability in mind.
For example, an API for user authentication or product listings can serve both Android and iOS apps simultaneously. Proper versioning and modular design make reuse easier and more maintainable.
What's the difference between public and private APIs in mobile app development?
Public APIs are designed for external developers and third parties to access, often with rate limits and documentation available online.
Private APIs, however, are used internally within an organization to connect services and apps securely. Mobile apps typically use private APIs for internal data operations and public APIs for services like maps or payments.
Is it necessary to host an API on cloud services like AWS or Azure?
While it's not mandatory, hosting APIs on cloud platforms like AWS, Azure, or Google Cloud offers scalability, monitoring tools, security layers, and performance benefits.
Small apps may start with shared hosting, but cloud infrastructure is recommended for production-grade mobile applications due to its reliability.
How do APIs impact the performance of mobile applications?
APIs significantly influence app performance. Poorly optimized APIs can slow down response times, increase battery usage, and affect user experience.
Factors like network latency, payload size, and backend server speed all contribute. Proper caching, lightweight responses, and async operations help enhance performance.
Can low-code or no-code platforms be used to create APIs for mobile apps?
Yes, several low-code and no-code platforms offer API creation tools, such as Firebase, Backendless, and Appgyver.
These are great for prototyping or simple applications, but for advanced business logic, custom development using traditional coding stacks is still preferred for better control, scalability, and flexibility.
Build Your API-Driven Mobile App with Developers.dev
Want to Power Your App with Fast, Secure & Scalable APIs? At Developers.dev, our experienced developers specialize in creating robust, high-performance APIs that fuel seamless app experiences.
From real-time data sync to third-party integrations, we design API architectures tailored to your app's exact needs. Let's build something powerful together!