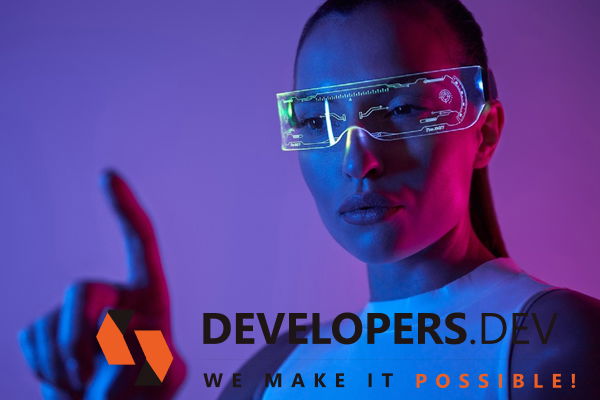
Programmers commonly use the Java Collections Framework (JCF), a collection of classes and interfaces for creating reusable collection data structures.
It makes Java programming one of its core features, providing interactivity among programmers as they store, retrieve and alter aggregated data sets. Careful consideration and implementation may result in code methods that are more effective, efficient and intuitive; hence, this article offers helpful benefits, core interfaces on utilizing collections wisely while emphasizing best practices and simplicity.
Java Collections
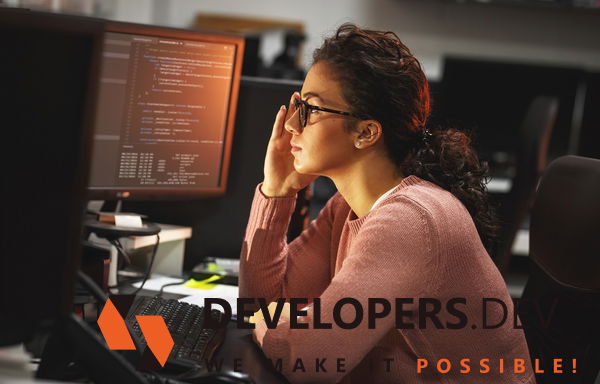
The Java Collections Framework (JCF) is an integral component of Java programming designed to manage groups of objects dynamically.
Compared with traditional arrays, collections provide greater flexibility for handling data dynamically - providing powerful utilities like dynamic sorting. This extensive framework provides a wide range of interfaces, implementations, and algorithms that can greatly improve programme speed and code efficiency.
Java Collections provide an architecture to store and manipulate groups of objects. Java Collections support many operations for managing data, such as searching, sorting, inserting new records into an existing one and even deletion.
Java Collection refers to any single unit of objects within its framework. It provides various interfaces (Set, List, Queue and Deque) and classes such as ArrayLists, Vector Linked List, Priority Queue HashSet, LinkedHashSet, TreeSet etc, for managing these collections of data.
Java Collections Framework comprises several parts, as shown here:
Interfaces
The Java Collections Framework interfaces offer the means of representing collections abstractly in an accessible data type format.
Java.util.Collection is the core interface for Collections Framework and should sit atop any hierarchy involving collections. This interface features some essential methods like size(), iterator(), add(), remove() and clear() that all collections classes must implement to operate effectively.
Other key interfaces in Java.util include List, Set and Queue Interfaces as well as Map which does not inherit from Collection; all four collections framework interfaces reside under this package in java.util.
Implementation Classes
Java's Collections framework offers implementation classes for core collection interfaces that we can use to build various types of collections in our Java programs quickly.
Important collection classes include ArrayList, LinkedList, HashMap, TreeMap, HashSet and TreeSet - these should fulfill most programming needs; but should anything unexpected arise we may extend them or create our own custom collection class as required.
Algorithms
Algorithms are useful tools that provide some common functionality like searching, sorting and shuffling.
Java Collections Framework Benefits
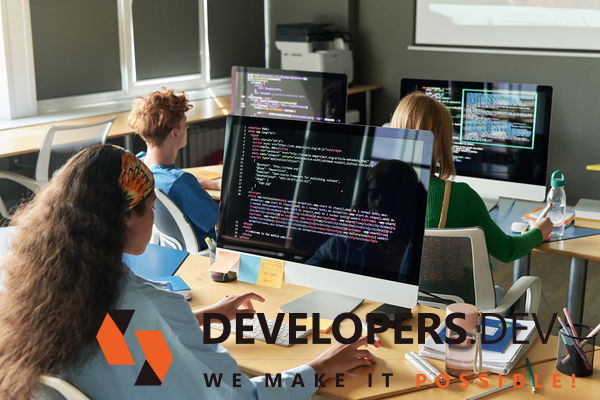
Here are some benefits of Java Collections Framework for its users:
- Reduced Development Effort: Spring Boot provides almost all commonly used collections as well as useful methods to iterate and manipulate data, so we can focus more on developing our business logic than designing APIs for our collections.
- Better Quality: Utilizing proven core collection classes enhances program quality over employing any homegrown data structure.
- Reusability and Interoperability Feature.
- Reduce maintenance effort since everyone knows Collection API classes.
Read More: Maximizing Java Development: Custom, Secure and Feature-Rich Solutions
The Java Collections Framework's Core Interfaces
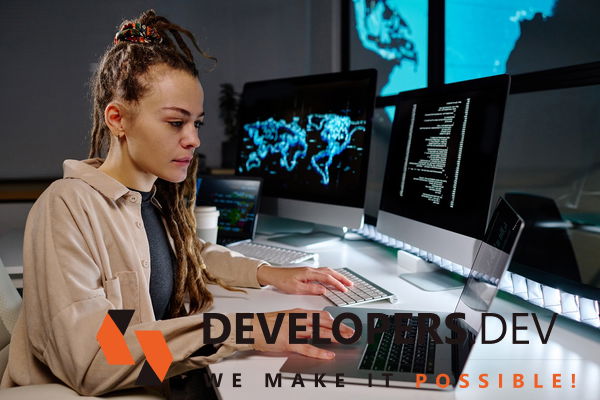
Java collection interfaces form the cornerstone of the Java Collections Framework.
All core collection interfaces, like public interface Collection are generic; to declare them we should specify their type in order to reduce runtime errors through type checking at compile time. This helps avoid runtime errors which might arise by mismatches between Object types contained by collections at compile-time vs runtime.
So as to maintain manageable collection interfaces, Java does not offer separate interfaces for each variant of each collection type.
When an operation that cannot be supported is invoked on one, an UnsupportedOperationException is raised from collection implementations.
Collection Interface
The Collection interface serves as the root of a collection hierarchy and represents groups of objects known as its elements.
However, no direct implementations exist on Java platforms for this interface. This interface includes methods that let you determine how many elements there are in a collection (size and isEmpty), check if a given object exists within it (contains), add or remove an element (add and remove respectively), as well as providing an iterator over said collection (iterator).
The collection interface also has bulk operations methods called containsAll, addAll, removeAll, retainAll, and clear that can be used to perform operations on every item in a collection as a whole.
ToArray methods serve as an intermediary between collections and legacy APIs that rely on arrays as input data.
Iterator Interface
This Interface offers methods for iterating over individual elements in a Collection, using its Iterator() method and retrieving an instance thereof using the iterator() method.
In Java Collection Framework terms, Iterators take over Enumerations's job of iterating elements through collections while permitting callers to add or delete items during iterations; Iterators implemented by collection classes follow the Iterator Design Pattern as part of this implementation.
Set Interface
A set is defined as any collection which cannot contain duplicate elements and this interface enacts mathematical set theory to represent physical sets such as decks of cards.
Java provides three general-purpose Set implementations: HashSet, TreeSet and LinkedHashSet. None of them allow random-access elements within their Collections - only an iterator for each loop can traverse these Sets' elements.
List Interface
A List interface provides an orderly collection that may contain duplicate elements and allows access by index. ArrayList and LinkedList, implementation classes of List, provide one way of realizing its capabilities.
List interface provides useful methods for adding an element at an index number, replacing elements based on index number and getting a sublist using index as its basis.
Queue Interface
A queue is a collection used to hold various elements before processing; beyond basic collection operations, queues offer additional insertion features.
Queues typically, though not necessarily, employ a first-in, first-out (FIFO) ordering of elements, with priority queues providing exceptions that use other measures based on comparator requirements or naturally ordered elements to rank elements accordingly. Whatever method is used, the head of any queue represents those elements that would be removed upon receiving a call to remove or poll request; all new elements would instead enter through its tail in case of a FIFO queue.
Dequeue Interface
A linear collection supporting element insertion and removal at both ends; deque is shorthand for "double-ended queue," often pronounced deck.
Most implementations do not place fixed limits on how many elements may fit inside; this interface supports capacity-limited deques as well. This interface defines methods to access elements on both ends of a deque, including ways to insert, delete or inspect elements.
Map Interface In Java
A map is an object that connects keys and values. A single key may only ever map to one value within its boundaries.
Java provides three general-purpose Map implementations: HashMap, TreeMap and LinkedHashMap. Map provides several basic operations: put, get, containsKey, containsValue, size and isEmpty.
ListIterator Interface
An iterator designed specifically to work with lists. Programmers may traverse in either direction while editing during iteration as well as access their current position within iterations.
Java ListIterators lack a current element; instead, their cursor position always lies between that returned by previous() and next() calls.
SortedSet Interface
A SortedSet is a Set that displays its elements in ascending order. Additional operations exist to take advantage of its ordering; therefore, it is used when working with naturally ordered collections such as word lists and membership rolls.
SortedMap Interface
This map keeps its mappings in ascending key order - analogous to SortedSet for sets - making them particularly suited for naturally ordered collections of key/value pairs such as dictionaries or phone directories.
Java Collections Best Practices
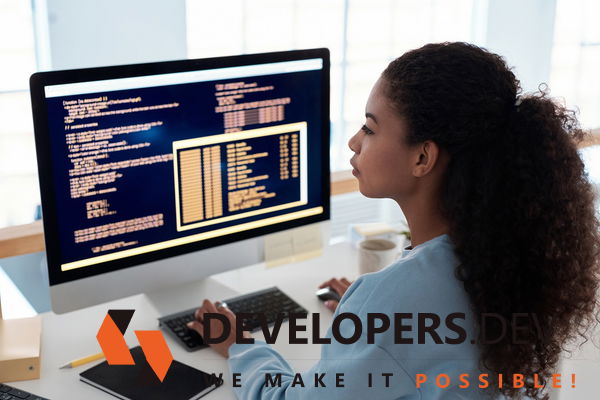
- Prefer Interfaces Over Implementations: When declaring collection variables, prefer interface types over specific implementation types for maximum flexibility and easier implementation switching if required. This also supports decoupled code from specific implementations which provides greater scalability if changing is ever required.
- Examine Beginning Capacity: To avoid repeated resizing or rehashing operations that can impair performance, consider the beginning capacity of classes such as ArrayList and HashSet.
- Utilize Collections Utilities: Java provides utility classes like Collections and Arrays, which offer various ways of manipulating collections, such as sorting, searching and converting arrays to lists. Utilizing such utilities can streamline common tasks while improving code readability.
The Java Collections Framework is an invaluable tool that, when properly utilized, can provide solutions to many programming challenges.
By understanding its core interfaces and selecting suitable implementations while adhering to best practices for using it, developers can write more efficient, cleaner and maintainable Java code.
Want More Information About Our Services? Talk to Our Consultants!
Conclusion
The Java Collections Framework is an indispensable asset to developers working in Java development services, providing efficient data management and manipulation.
Through this article, we covered essential aspects such as selecting appropriate collections, using generics for type safety, understanding these concepts enables programmers to write more effective yet maintainable Java code while adopting these practices and exploring further will greatly increase coding proficiency and application performance.