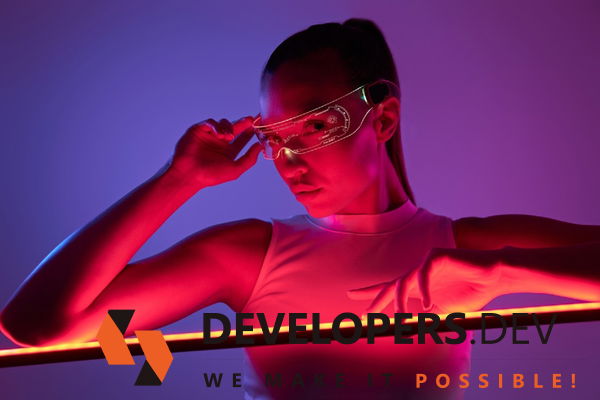
No matter the programming language you choose, to keep your software secure it must adhere to current best practices and adhere to standards set forth.
Java is no different: vulnerabilities remain even with its wide usage. In order to provide a foundation for safe Java development, we've put together this set of rules; by adhering to them, you will soon have software that withstands vulnerabilities encountered during production and becomes immune to security risks.
In this blog, we delve into the securities of Java development, exploring its best practices and more. Dive in for valuable insights.
Java Development
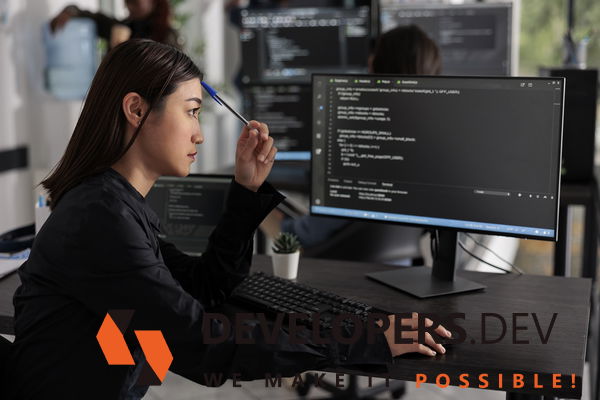
What Is Java Development? Java has long been associated with backend development due to its focus on logic, data and functionality in an app or website.
Backend developers typically interact with databases, servers and APIs not visible directly to end users - though some projects have attempted bringing Java closer directly towards users as frontend solutions - though rarely used this way.
Java offers web administrators several development tools - Servlets, JSP and Struts among them - which enable rapid website development.
These allow them to quickly develop backend logic, process data efficiently across database connections and handle HTTP requests and responses efficiently - as a result many consider Java primarily suitable for backend functions of websites.
Java Has Vulnerabilities In Its Security Architecture
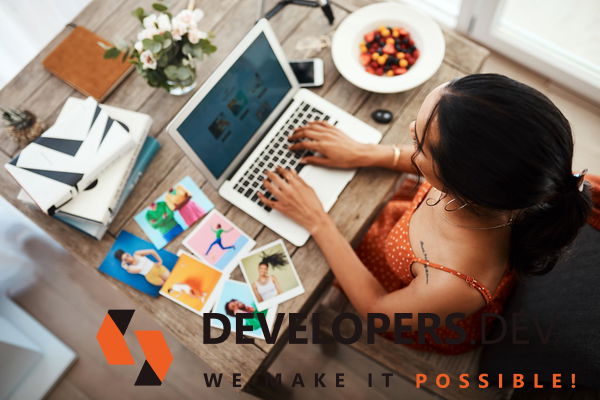
Java is widely recognized for its rigorous, systematic, static-typed and security-conscious development paradigm.
It remains popular with large organizations where security and consistency of processes are of great significance for their development teams and security processes.
Unfortunately, however, like any programming language, there can still be security vulnerabilities introduced into a Java development cycle due to developers using libraries that do not adhere to the latest vulnerability lists and building components with known flaws that weren't carefully reviewed before being included into a build process.
Here are the key Java security vulnerabilities you should keep in mind while developing software applications.
Cross-Site Scripting Information Is Stored
Stored cross-site scripting occurs when an attacker injects malicious script directly into web applications without needing user interaction or input in order to gain access to sensitive data.
Such attacks pose a great danger as their malicious code could gain entry through any means necessary, allowing hackers access to sensitive information that would normally require interaction from users or interaction from them directly.
Social media sites are particularly susceptible to stored XSS attacks due to the nature of user engagement; users often post and interact, providing opportunities for malicious code injection without user knowledge or consent.
XSS attacks, often known as webworms, involve users encountering content with offending elements that executes in their browser and results in stolen cookies, account information or additional functions being exploited through account impersonation. Furthermore, this code also has remote code execution properties.
Reflect Cross-Site Scripting
This type of XSS attack uses malicious scripts activated via links, sending users away and stealing their session authentication to modify user data or gain entry to accounts.
User input validation can help reduce risks from this form of cross site request forgery, especially as reflected XSS attacks rely heavily on using users' browsers as targets to execute scripted attacks on sites.
SQL Injection Attacks
SQL injection attacks involve injecting malicious SQL statements into data requests in order to gain entry to databases and gain entry.
This could cause sensitive data or scripted content that changes them on servers being returned, with additional uses as potential means for deceptively sending untrusted material under false pretenses.
SQL injection can lead to access of sensitive data and breaches in privacy, data loss or corruption and possibly locking you out of your own database.
One effective defense against SQL injection attacks is through server side validation: this helps block unwanted characters such as spaces and quotation marks from coming through and creating security breaches.
Code Injections
Web applications that accept user input may be at risk from code injection attacks, where user data leads to unexpected side-effects in how your server-side Java app runs or returns data.
Forms are two-way processes between users and databases; when these transactions don't go as intended, however, web security vulnerabilities could arise as a result. Code injection is an increasingly prevalent trend and is generally easy to carry out.
OS Command Injection Attacks
Operating system command injection attacks are security vulnerabilities that enable attackers to execute shell commands against the web server that hosts your application.
PHP is most vulnerable to command injection attacks due to its habit of calling bash/cmd by default, while Java performs fork() of commands as child processes with given arguments passed in as parameters.
When implemented properly this approach should work without issue, but legacy code could introduce vulnerabilities which allow attackers to exploit shell command injection vulnerabilities and execute commands remotely.
Connection String Injection
Connection strings are used to link an application and data sources such as relational databases, LDAP directories or files.
An attacker looking to gain entry to a database needs four parameters in order to inject their connection string: data source, initial catalog, user ID and password.
Connection string attacks occur when an outside actor gains access and injects parameters using semicolons as separators into connect strings, creating malicious software code or access to resources on an internal server or network.
Read More: Transform Your Business with Java App Development
Best Practices For Java Security
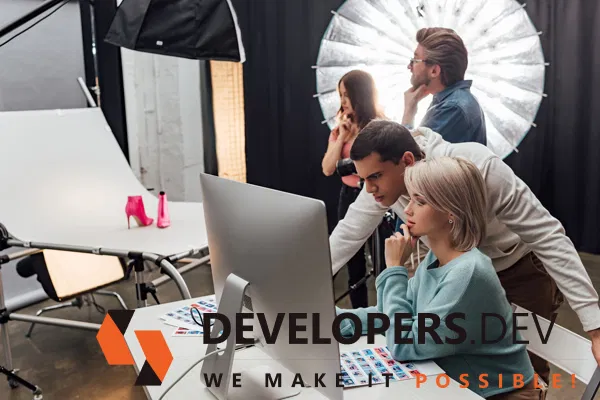
Here are some best practices for Java Security:
To Isolate Internal Code, Use Modules
One of the many things we admire about Java is its modules system and access modifiers; taking full advantage of them can lead to more secure Java code.
Java modules are collections of packages intended to be reused. A module encases classes and interfaces within its packages except for public classes/interfaces that have been exported by its parent module - meaning code outside can access only these public classes/interfaces via code outside its packages while accessing other public classes/interfaces that don't belong to an exported module will still require code within said package to access.
Packages not exported are hidden to code outside.
Declare a module so that packages containing published APIs are exported while packages supporting implementation aren't.
This ensures implementation specifics won't become vulnerable to malicious activities and expose implementation details that might lead to breaches in security. Likewise, all exported packages should be reviewed to make sure no security-sensitive classes or interfaces have been exposed - adding extra exports in future should not create compatibility problems.
Don't Overcomplicate Your Java Code
As with any program you develop, adding layers of complexity increases the potential for vulnerabilities to arise.
When doing so, Java's static typing and mature language conventions become less beneficial; here are a few techniques to keep your code simple in the interests of security:
- Use access modifiers to conceal implementation details from end-users by employing private settings whenever possible.
This way you're protecting both end-user privacy and your own implementation details from exposure.
- Develop modularity and organize your API.
- Use Developers to detect all the latest Java vulnerabilities present in your software release.
Use Libraries That Are Proven And Popular
Open-source libraries have long been relied upon to speed development and foster innovation. However, selecting popular and regularly updated libraries should always be your goal.
Popularity can help spur regular updates, while it also means there will be more eyes watching a project at any one time - meaning vulnerabilities could more quickly be discovered and remedied before pushing your code out to production. Utilizing trusted libraries and open-source code will reduce security risks within your software application and help keep its Java codebase compliant with industry best practices for security.
Check Your Exceptions And Handling Procedures
Errors may arise because of bad inputs, logic errors or misconfiguration. While it would be ideal to prevent or avoid situations that cause such events in the first place, secure Java code should always assume an unexpected exception may appear at any moment.
If a method call results in an exception, the caller must decide between handling or propagating it:
- Handling an exception involves catching it and performing corrective, cleanup, or fallback actions as necessary before continuing as normal so as to shield the caller's own callers from experiencing error conditions.
- Propagating an exception exposes an error condition to its caller, giving that caller the choice between handling or propagation. Propagation could involve catching, wrapping and rethrowing it or could involve no explicit actions taken at all; in either event, runtime unwinds its current call frame and delegates to the caller's caller for handling decisions.
Serialization Remains An Issue
Oracle is in the process of eliminating serialization from Java entirely. Still, while serialization remains an important feature, vulnerabilities must be taken into consideration.
Any application using serialized Java objects could potentially expose itself to vulnerabilities; open-source libraries do not exempt themselves; always remember whether data you receive from an API or library utilizes serialized objects when considering these factors.
Inheritance also causes serialization problems as it's easy for an inherited subclass to unwittingly implement a serializable interface, leading to serialization problems in subsequent subclasses.
Double check your documentation so as to prevent potential serialization problems arising due to inheritance.
Keep serialization out of any security-sensitive classes as much as possible, too. Serializing classes effectively creates an open interface to all fields within it - something not recommended when used for purposes of protection.
Lambdas require equal scrutiny. Functional interfaces pose similar threats of becoming public should they become serialized.
Filters may be applied to serialization in JDK 9 or open-source equivalents; standard practice dictates validating input before it's used, and serialization filtering allows classes to be validated prior to deserializing them.
Overall, when possible it's best to avoid serialization; otherwise filter where needed and try to reduce use of serialization and deserialization as much as possible.
Hash User Passwords
Although this may seem obvious, be sure to implement a salted hash when protecting user passwords with SHA-2 hashing algorithms - plaintext storage opens you up to malicious activity and could compromise all user information stored therein.
Hash passwords to protect data in any context or use scenario.
Do Not Log Sensitive Information
Data theft has become a serious risk in today's cybersecurity environment. Sensitive information, including credit and debit card numbers, bank account details, passports, and social security numbers, could all become available to criminals for gainful gain.
Log files might tempt you, as they provide easy ways of recreating technical issues or tracking data across an org; but before doing so it's essential that context within the Java app itself be taken into consideration and this data kept accessible when stored as logs.
Administrators and staff shouldn't have access to sensitive data either; therefore, it is vital that secure code be created that prevents this information from becoming accessible within internal teams as well.
Want More Information About Our Services? Talk to Our Consultants!
Conclusion
Although many of these principles pertain specifically to Java applications, following them will ensure your software remains safe and secure regardless of which programming language it was written in.
Always consider security during design stage reviews as well as code reviews of your software; look out for vulnerabilities within its Java codebase while taking advantage of available APIs and libraries provided by Oracle Java Secure Coding Labs.
Developers will assist in keeping your Java codebase safe with up-to-date language implementation features and static and dynamic analysis tools that protect it against SQL injection, Cross-site scripting and serialization issues.
Rest easy knowing Developers have you covered for maximum protection for Java development services.