
With Java Concurrency, you can harness parallel processing and multithreading to boost your apps.
No matter if you're just getting started or an experienced programmer - this blog is here to get your feet wet in concurrent programming and help guide your skills towards high performance coding. Get cozy as we explore Java's expansive APIs together for an unforgettable adventure into concurrent coding.
Concurrency: What It Is?

Concurrency, or the capacity for software programs to simultaneously complete multiple tasks at the same time, is used by top Java development businesses as it helps optimize an app's overall performance and responsiveness while making optimal use of system resources.
As all the core classes and interfaces necessary to support multithreading in Java concurrency - such as Thread, Runnable classes in java.util.concurrent are contained within standard libraries of the language, there shouldn't be significant variations across frameworks in their multithreaded capabilities.
Java Multithreading
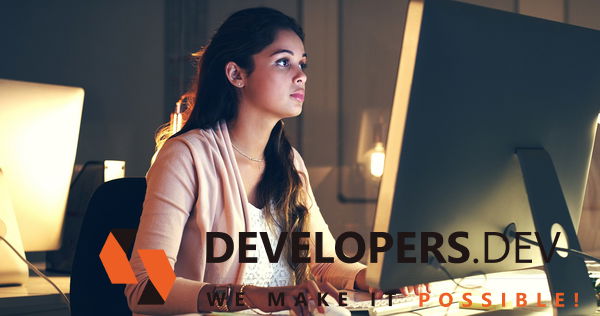
What is Multithreading? Multithreading refers to an approach in programming in which an application features multiple threads of execution within itself.
Achieving concurrency using Java can be done using multithreading; alternative methods could also include Asynchronous programming, Event-Driven Programming or multiprocessing as a means. A "thread" refers to any single stream of tasks that a computer's processor can complete independently of other threads running at once.
Benefits Of Multithreading
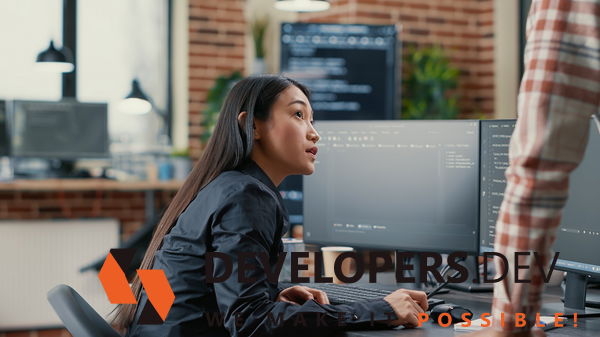
- More units of information can be processed within a specified amount of time with greater throughput, creating better responsive apps with smoother interactions for users and giving the illusion of multitasking capabilities.
- Increased resource efficiency: When it comes to processes, creating threads typically costs much less than starting from scratch.
- Web servers that rely on threads instead of new processes when responding to queries from users use far fewer resources overall.
Challenges Of Multithreading
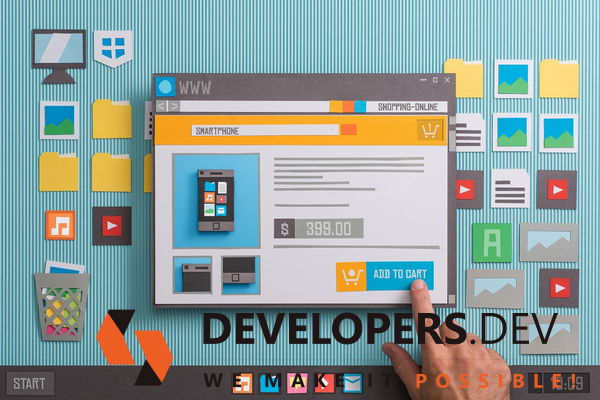
- Locating Bugs Can Be Difficult: Operating Systems will dictate which threads run first and when. Their results may not always be predictable. Maintaining Multithreaded Code Gaining more complexity means increasing costs associated with code maintenance costs incurred from maintaining multiple threads simultaneously, plus their separate upkeep expenses can mount quickly over time.
- Increased System Demand: Each new thread increases system demands in terms of memory usage and CPU cycles to maintain records, as well as time spent switching contexts between processes. Furthermore, processors handling five threads concurrently require extra registers in order to track each process's information while it runs alongside its peers.
Why Should You Use Java Concurrency?
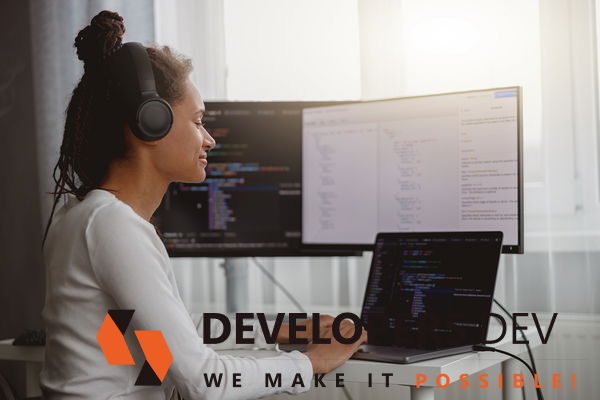
Here, we have outlined the significant reasons for utilizing Java Concurrency:
- Enhance Performance: Concurrency allows businesses to break complex processes down into small chunks that can be completed more rapidly, significantly speeding up application development by taking advantage of multi-core CPUs available today. This has an enormously positive effect on performance.
- Concurrency Can Increase Resource Utilization: Concurrent use of system resources maximizes efficiency, increasing resource utilization. A system can maximize consumption and system efficiency by permitting concurrent processes to run at once while eliminating single thread blocking through providing asynchronous I/O operations.
- Concurrency Increases Responsiveness: Concurrency can ensure an interactive application does not become inoperable and enhance user experiences by handling inputs/updates from one thread while a different thread completes computationally demanding operations in parallel.
- Simplified Modeling: Concurrent programming methods offer more efficiency and simplicity when dealing with situations involving concurrent entities that naturally occur within a problem domain, such as simulations or game engines. This practice is known as simplified modeling in general.
- Sturdy Concurrency API: Java's robust concurrency API offers many flexible and comprehensive features such as thread pools, concurrent collections and atomic variables to make its flexible concurrency API useful and practical for writing concurrent code. These tools help reduce common concurrency issues as they facilitate writing.
Concurrency Drawbacks
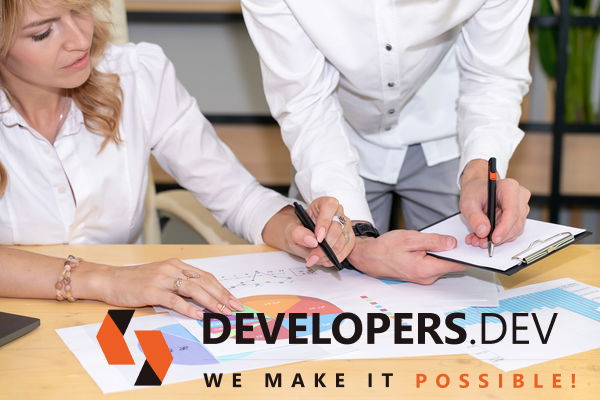
Concurrent programming should not be taken lightly by novice programmers. Concurrent coding adds another level of complexity and presents unique challenges such as thread safety assurance, managing synchronization and avoiding deadlocks - so before getting started, it is wise to consider some key considerations before jumping in.
Here is some guidance:
- Complexity: Writing concurrent programs may prove more complicated and time consuming than creating single threaded ones, making developers even more adept at understanding issues such as synchronization, memory visibility, atomic operations and thread communication than when designing single-threaded programs.
- Debugging Challenges: Debugging concurrent programs can be complex due to their non-deterministic nature and unpredictable behaviors, often creating race conditions or deadlocks which make reproduction and resolution of these instances challenging.
- Error Potential: Improper concurrency management may result in race situations, deadlocks and thread interference which are among many errors which arise as a result. Unfortunately it could be difficult to quickly recognize and correct such problems.
- Resource Contention: When multiple threads compete for access to one resource in an ineffective concurrent application, resource contention occurs, and performance diminishes accordingly.
- Overhead: Thread creation and management can lead to increased CPU and memory use on your computer, potentially depleting resources quickly or leading to less than ideal performance. Ineffective management could result in wasted effort or decreased resource use altogether.
- Elaborate Test Cases: Due to thread execution's unpredictable and non-deterministic behavior, testing concurrent programs is an often difficult process.
Read More: Mastering Java Development: A Comprehensive Guide for this year
Deadlocks
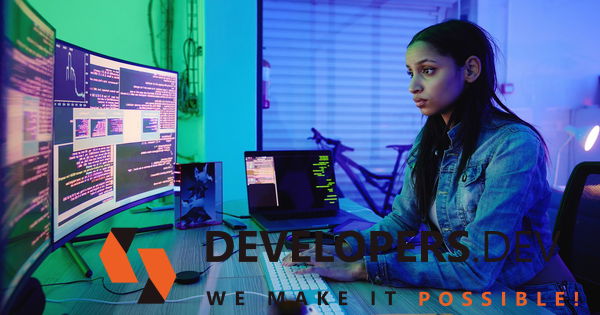
Deadlock occurs when multiple threads become frozen, waiting on one another to release resources or perform specific actions; as a result, neither thread advances further and remains imprisoned forever in an intractable situation.
Let's consider two locks (LockA and LockB), two threads (threadA and threadB), and two attempts by ThreadA at unlocking LockA. Should they fail, ThreadA will instead move onto LockB, while ThreadB makes its first effort toward LockA first before trying for LockB.
ThreadA has obtained lockA and is waiting on lockB, while ThreadB is attempting to acquire it by holding lockA. Since ThreadA holds both locks simultaneously in this instance, threads will never receive either, creating what we refer to as deadlock conditions - to avoid these in future discussions, keep in mind these points:
- Outline an effective plan for the acquisition of resources.
- Each thread must follow an agreed upon sequence when making resource requests.
- Avoid using synchronized blocks and lock nesting. In our previous example's deadlock situation, threads couldn't open one lock without also getting hold of both locks simultaneously resulting in deadlock.
- To address this, lock nesting should also be avoided in order to maintain efficiency of operation and ensure threads can release one without simultaneously unlocking both.
- Keep an eye out to ensure threads do not acquire multiple resources at the same time, which reduces deadlock and circular dependency issues.
- Set timeouts when gathering locks or resources.
- A thread may release all locks it has obtained within its allotted period if it was unsuccessful in getting hold of any.
Java Concurrent Collections
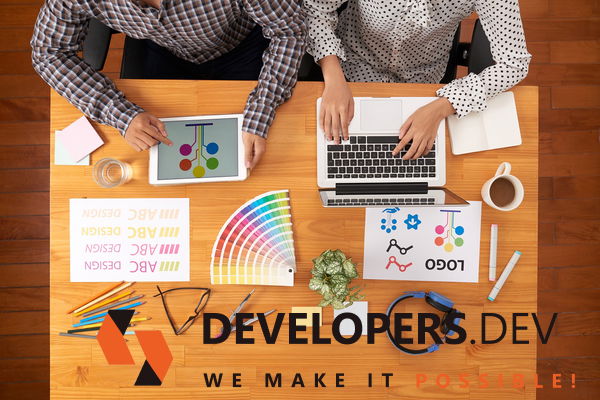
Access is made concurrent via several thread-safe concurrent collections found within Java's "java.util.concurrent" package.
- ConcurrentHashMap: ConcurrentHashMap provides an alternative, thread-safe HashMap experience by offering retrieval and update techniques tailored specifically for retrieval and atomic update processes such as PutIfAbsent(), delete() and replace() which atomically execute actions while eliminating race situations.
- CopyOnWriteArrayList: Instances where another thread attempts to edit an array list while another one reads or iterates over it could lead to inconsistent read operations and result in ConcurrentModificationException. CopyOnWriteArrayList can help by copying its full contents every time an array is changed, allowing us to refine new copies while iterating over old ones. CopyOnWriteArrayList's thread-safety method carries with it some costs; adding or removing members requires copying an array. Because of this, this solution works better in situations in which reads occur more frequently than writes.
Java Concurrency Alternatives
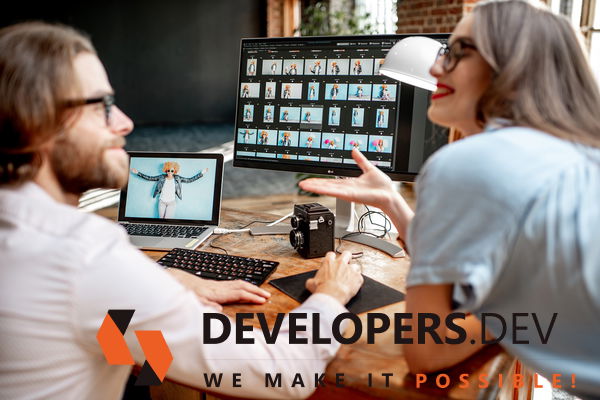
Java concurrency can be an excellent option when building OS-independent, high-performance applications, but other choices exist as well.
Here are a few alternatives you might wish to explore: Google created Golang (also referred to as Go), a statically typed programming language frequently utilized in Java development services.
Go's effectiveness and efficiency when managing multiple tasks simultaneously are highly praised by reviewers; its lightweight threads controlled by Go runtime known as goroutines are essential in its prowess as concurrent programming solutions as they make concurrent programming both straightforward and incredibly effective.
Many Scala development companies favor Scala due to its JVM compatibility, elegantly blending functional and object-oriented paradigms.
Scala's vast Akka library stands out as its highlight feature; specifically designed to manage concurrent operations using Akka's Actor model of concurrency it offers less error prone yet user friendly solutions compared to thread based concurrency solutions.
Python provides concurrent programming libraries like Asyncio, multiprocessing and threading which give developers the ability to manage concurrent and parallel execution efficiently and with greater ease than with many other languages; but be wary of Python's Global Interpreter Lock (GIL), which may limit threading effectiveness when running CPU-intensive operations.
The functional programming language Erlang/OTP was specifically developed to build highly concurrent systems. OTP serves as middleware that offers libraries and architectural guidelines to make this possible.
Conclusion
Java concurrency provides developers in an age of multi-core processors with a useful tool that enhances program performance through parallel processing and multithreading.
As it utilizes strong APIs, concurrent programming is reliable and efficient - however, it presents its own set of challenges; shared resources must be managed carefully in order to avoid race situations, deadlocks and thread interference problems that arise from concurrent programming in Java - but its benefits to application performance often outweigh these costs.