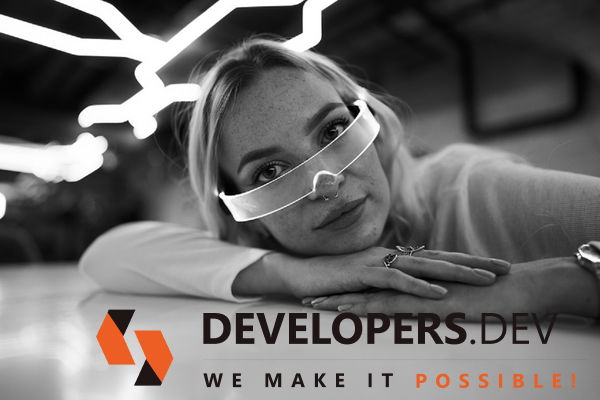
Design patterns are a collection of tried and tested solutions for issues commonly seen during object-oriented software development.
Experienced software developers have come up with these patterns after many rounds of trial-and-error testing over many years.
This guide will introduce the core Design Patterns used in Java that you should know. Gradually, you will progress from an introduction that covers the why and how of Design Patterns into practical examples to understanding each design pattern through hands-on experience.
Design Patterns
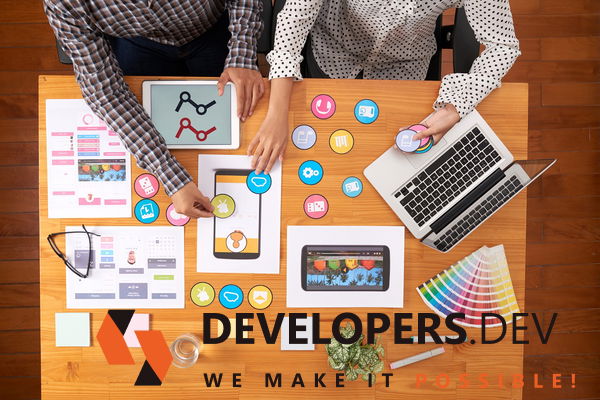
What are Design patterns? Design patterns are repeated solutions for software design problems used in software engineering.
While not an immediate code solution, design patterns provide a framework or model that may be applied across contexts for problem-solving purposes. They have become very popular among software developers as a reliable method to solve common software issues.
Design patterns should not be seen as simply solutions to problems but as templates that can be customized according to individual problem statements.
Each design pattern serves as a blueprint that can help solve design-related problems at any point during development of software modules or can even be embedded at later stages in any process. They offer language-independent strategies which make your code flexible, manageable and reusable - something every good developer aims for in code.
Why Does Java Need Design Patterns?
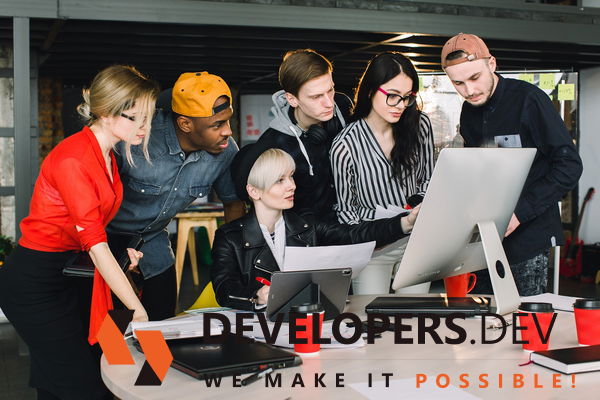
Design patterns have long been part of a software developer's toolbox to solve design challenges, so why are we discussing them specifically in terms of Java development? Java is built around design patterns; for instance, Swing uses Observer Patterns, while input and output stream readers utilize Adapter patterns.
Therefore, to properly utilize Object-Oriented Java, it's vitally important that you become well versed with Design Patterns; they offer general solutions to common design challenges that need solving consistently over time. Let's go over some features of Design Patterns found within Java;
- As they are template solutions, these can easily be reused.
- Experienced software developers have designed these solutions that have stood the test of time, creating solutions with proven success rates over years of use.
- They foster transparency in application design.
- Design patterns enable more efficient collaboration among developers by serving as general solutions for various problems.
The Benefits Of Design Patterns
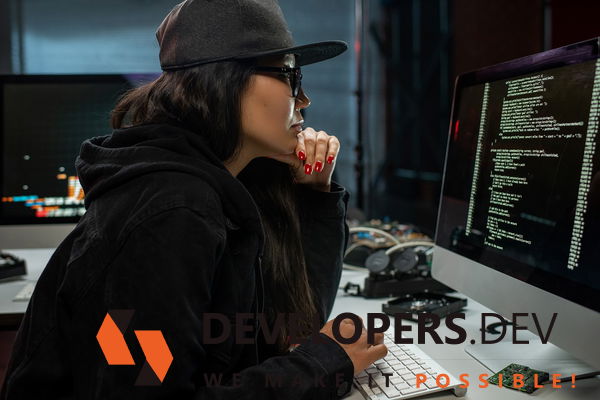
Here are some benefits of Design patterns:
- These blocks can be reused across various projects.
- Solution providers deliver services and tools that help define system architecture.
- They capture experiences gained in software engineering.
- Design patterns provide clarity to an application's development process.
- Solutions developed by expert software developers are tried-and-tested solutions.
- Design patterns don't offer absolute solutions to problems; rather, they offer clarity into system architecture and increase the chances of creating an improved solution.
When Should Design Patterns In Java Be Utilized?
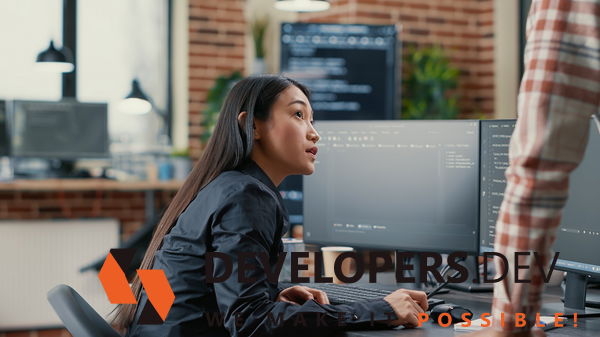
Design Patterns provide developers with a valuable way to quickly come up with well-structured solutions using information that they already possess, connecting their project requirements to an already proven approach for similar problems.
Employing design patterns during analysis and design phases during software development life cycles would therefore be a wise decision.
Java Design Pattern Categorization
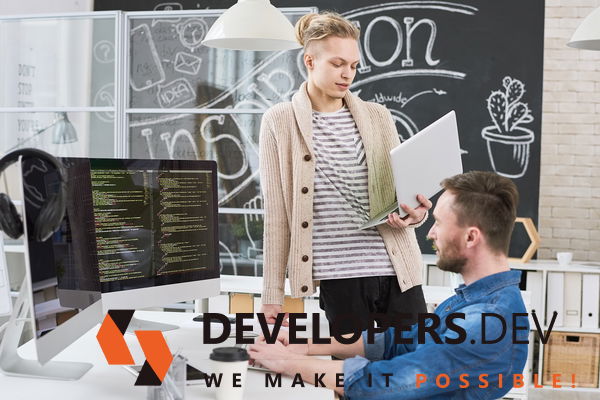
Design patterns can generally be divided into the many components, read about categorization of Java Design Pattern:
Creational Design Patterns
Creational Design Patterns (CDPs) are often employed during class initiation procedures and deal with creating objects.
There are various classes of Creational Design Patterns:
- Factory Pattern: Here, we define an interface or abstract class and allow subclasses to decide who may instantiate it.
- Abstract Factory Pattern (AFP): defines an abstract class or interface without specifying concrete subclasses; that is, it allows a class to become its factory of classes - making this pattern one step up from Factory Pattern.
- Singleton Pattern: It allows you to define a class with only one instance that is available globally by all classes; using static keywords allows for this purpose and creates variables and methods accessible across classes.
- Prototype Pattern: According to this method, when creating new objects is costly or time consuming, we may clone existing ones and customize them according to our needs.
- Builder Pattern: It allows for stepwise object construction using simpler ones and provides clear definition of its construction process.
- Object Pool Pattern: An object pool is a container that houses numerous objects and allows us to reutilize expensive pieces by pulling one out and returning it later. By keeping everything under one roof, we can reuse expensive objects more often.
Structural Design Patterns (SDPs)
Structural Design Patterns typically focus on class/object relationships by recognizing them to simplify complex structures.
There are multiple subcategories within them as well.
- Facade Pattern: It can help conceal the complex functionalities of a subsystem by consolidating all complex interfaces under one simple, higher-level interface.
- Bridge Pattern: This pattern allows for the separation of function abstraction from its implementation so both can vary independently of each other.
- Composite Pattern: It allows you to build an ordered hierarchy of classes containing complex and primitive objects, providing flexibility within its structure while making adding new functionalities much simpler.
- Decorator Pattern: Composition offers an alternative approach for adding or expanding functionalities at runtime.
- Adapter Pattern: According to this pattern, an interface from one class may simply be modified and converted to meet client-requested changes.
- Flyweight Pattern: It is an approach which involves using existing objects until none match, at which time a new object needs to be created.
- Proxy Pattern: To implement proxy patterns, we create another object that performs similar functions; this enables us to conceal sensitive information from original objects.
Behavioural Design Patterns (BDPs)
Behavioural Patterns typically involve interactions among objects and ensure their free communication while remaining loosely bound together.
Further classification includes;
- Chain Of Responsibility Pattern: It specifies that every request be sent through an ordered series of objects so that if one doesn't act on its request promptly, it can automatically pass to another in line.
- Strategy Pattern: It encases each class's functionality within itself to simplify adding new behaviors or expanding existing ones.
- Interpreter Pattern: It can be used for parsing expressions defined with rules known as grammar.
- Iterator Pattern: Used to access elements sequentially within an aggregate object.
- Mediator Pattern: It offers a mediator class to manage complex communications among classes.
- Memento Pattern: This pattern emphasizes saving a copy of what has changed during any transition in order to undo any mistakes, in case failure should arise.
- Command Pattern: Used to differentiate an object that initiates an operation from those that actually execute it, the Command Pattern is designed to separate them effectively and clearly.
- State Pattern: It emphasizes creating objects for every state.
- Observer Pattern: This design pattern establishes an object dependency so it can easily update in case of changes to one or both objects.
- Template Pattern: To avoid duplicative work and minimize redundancies, this pattern creates separate classes to house similar functionalities.
J-EE Design Patterns
J-EE Design Patterns focus specifically on providing solutions to enterprise edition-based frameworks like Spring.
Their offerings can further be classified as MVC Design Pattern, Dependency Injection Pattern, etc.
Read More: Maximizing Java App Development: Scalable, Secure Solutions
In Java, How Can One Create Design Patterns?
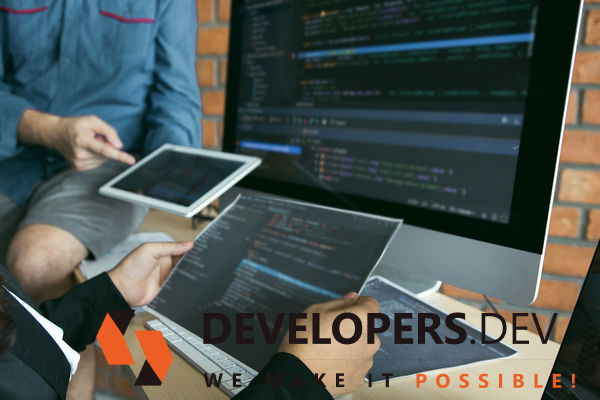
You can develop the desired Design Pattern by utilizing the numerous features and implementations that Java offers.
Based on your specific requirement, you can utilize interfaces, abstract methods or classes and structure them accordingly; additionally it also offers functional interfaces so that classes may be declared and defined explicitly.
Utilization Of Design Patterns In Real World Cases
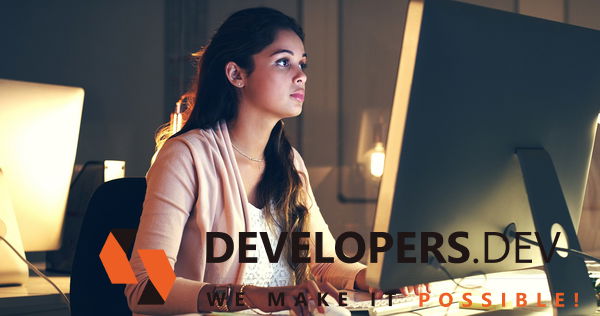
Imagine this. An example of a Factory Design Pattern is an abstract class named Cellular Network, which has three abstract methods for various pay-per-minute plans and is used to construct a network interface.
Furthermore, subclasses representing these plans could further define any of those abstract methods found within it based upon individual requirements - this would also constitute Factory Design Pattern usage.
Let's consider another scenario. Let's assume multiple users have registered on a website which displays cricket scores, and they all want to know when Kohli scores his next century; consequently, some might register as observers so as to receive notifications as soon as it happens - this example illustrates an observer pattern where multiple users become observers for one event at the same time.
Future Trends Of Design Patterns In Java
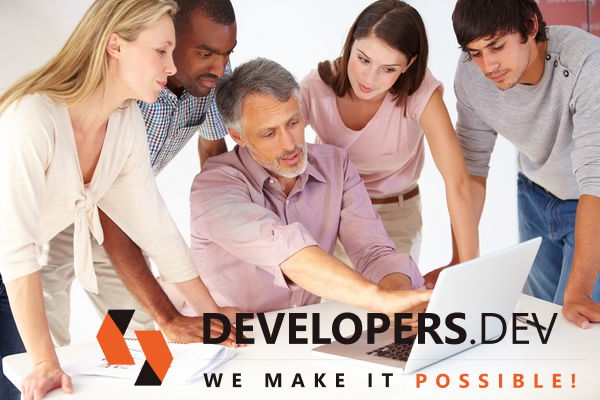
Design patterns in Java play an invaluable role in software development. Here are a few areas in which design patterns in Java stand out:
- Reusability Of Code: Design patterns provide an effective means of codifying common solutions to software design challenges, making it simpler to repurpose these solutions across projects while saving developers both time and energy.
- Maintainability: Design patterns provide a structured and consistent approach to software design, making it simpler and faster for developers to understand and modify code over time. This approach may reduce maintenance costs while increasing the lifespan of systems.
- Collaboration: Design patterns provide developers with a shared language and understanding to collaborate effectively on software development projects, helping facilitate better team communication and cooperation between team members.
- Design Patterns Can Enhance Software System Performance: Design patterns provide solutions that help enhance software system performance by offering optimized answers for common issues, like memory waste reduction. One such pattern, known as Flyweight, helps minimize an app's memory footprint by sharing objects across instances.
- Scalability: Design patterns can play an invaluable role in increasing software systems' scalability by offering solutions that are modular and extendible - for instance, Factory patterns can help.
Want More Information About Our Services? Talk to Our Consultants!
Conclusion
The process of using design patterns in Java may differ depending on both its usage and project needs. We discussed Design Patterns in Java in this article, how design patterns can help streamline solutions by using standard template solutions from previous problems as templates to solve new ones quickly and.
Furthermore, we covered their different categories, the benefits of their usage with real-world scenarios, when they should be them and some real-life use cases as well for more information regarding Java development services.