You will be happy to know that, yes, it is possible. Python is a great way to build Android apps. Another interesting fact about Python is that it is more complex and much easier to use on Android than Java.
This article will discuss how to use Python to create Android apps. We will then review the best tools and IDEs for Python to develop Android apps.
The post will conclude with the pros and cons of developing Android apps using Python.
What is Python Programming?
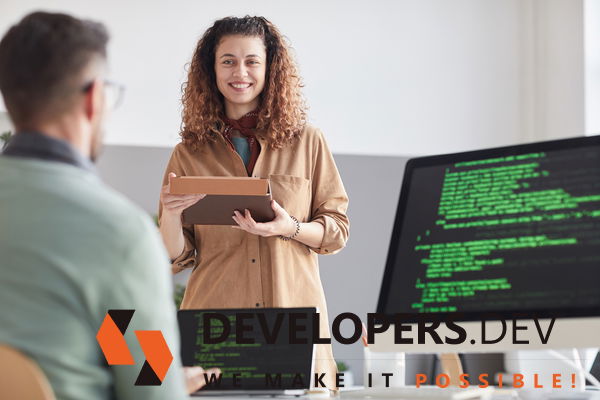
Python is a dynamic, object-oriented programming language. Python is a high-level programming language with dynamic semantics and built-in, high-level data structures.
Python can also be used to link existing components. It is easy to learn and reduces maintenance costs. Python promotes modularity in programming and the reuse of code.
The interpreter and the standard library in its binary or source form can also be distributed freely.
Why Choose Python?
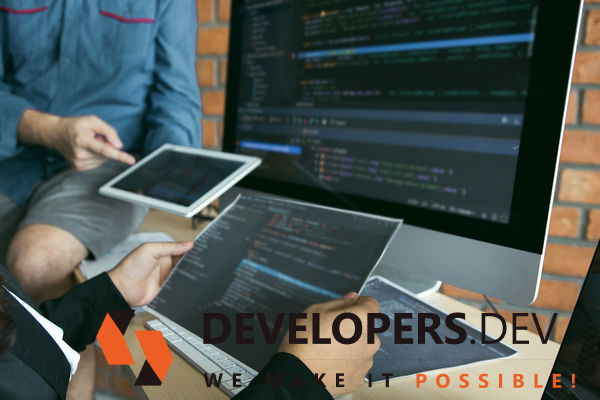
Python is a popular programming language because it increases productivity. The cycle of edit-test/debug is quicker as there is no compilation.
The most important thing is that Debugging Python applications is a simple task. A bad input or bug never causes segmentation faults.
The interpreter will instead discover an error and raise an exception.
It then prints out a stack trace. According to Analytics Insights Python has more than 29,000 stars on Github.
Source-level debugging allows you to inspect local and global variables. You can also set breakpoints and evaluate arbitrary expressions.
Python is the only language used to write this debugger, a testament to its introspective powers. The fastest way to debug your program is by adding a few statements of print to the source code.
It is simple to use and effective.
Follow These Steps To Install Python On Your Pc
- Download and install the Python installer by selecting the Python version.
- Install Python Executable Installer.
- Wait for the process to finish.
- Installing Python on Windows: Verification.
- Run Python
- Check that the Pip is installed.
Python Shell
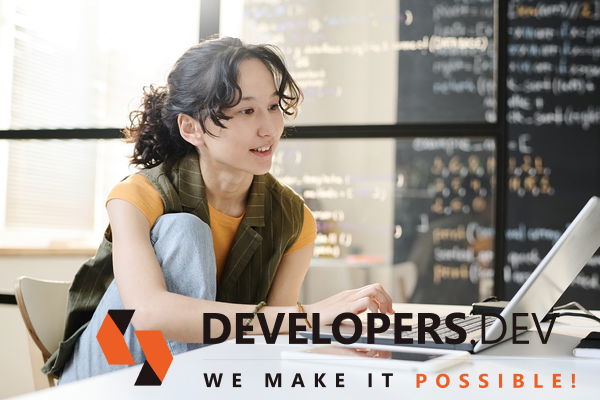
Python has a shell to run a Python command and then display the results. Python Shell, or REPL, stands for Read, Evaluate Print and Loop.
Python Shell reads complete commands, evaluates them, and then prints out the results. It loops back around to reread.
Python Shell is easily installed by using a Google extension.
What are the Basics of Python Programming?
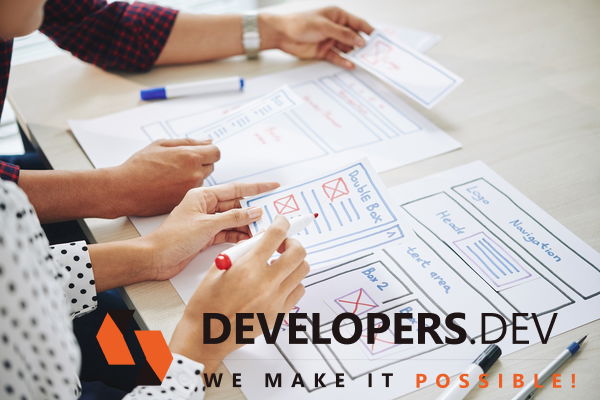
We will start by learning the basic concepts of Python. You can write a simple Python program after you understand the terminologies.
1. Fundamentals:
- The basic syntax of Python.
- This section explains the creation of concise, meaningful variables.
- Learn about the string and a few bais operations.
- Booleans are the Boolean type of data, which includes falsy or truthy values.
- This tutorial shows how constants are defined in Python.
- Comment: Learn how to add notes to your code.
- Conversion of type: This lesson will teach you how to change a value from one type into another. Converting a string into a number.
2. Operators:
- Compare two variables: you will learn how to use the comparison operators.
- How to use logical operators for multiple conditions.
3. Control Flow:
- Learn to run a code block depending on the condition.
- The Python ternary operators will help you to make your code concise.
- Break: Learn how to break a loop early.
- This shows how you can use the "pass" statement to create a placeholder.
4. Functions:
- Python Functions This course will teach you to use and define functions and the Python language.
- Use keyword arguments to make function calls more clear.
- Python Recursive Functions. Learn how to create recursive Python functions.
- Show how to use docstrings for documentation.
5. List:
- Tuple You must be familiar with the tuple. This is a fixed list.
- Sort the list on the spot you can sort your list using this method.
- The unpacking list method guides you through assigning list elements across multiple variables.
- This article explains how to make a list from an existing list.
- Use the filter to select elements from a list.
- Understand the difference between iterables and iterators.
6. Dictionaries:
- The dictionary introduces the type of dictionary.
- The dictionary comprehension section explains how you can use the dictionary comprehension feature to build a new dictionary using an existing one.
7. Sets:
- Sets this article explains a set and how you can manipulate its elements.
- It shows how to combine two sets or more using the Union Method.
- This tutorial shows how to use the set intersection or intersection methods of two sets.
- The symmetric method is used to identify the difference between sets.
- It checks whether a given set is a subset of another.
8. Exception Handling:
- It shows how you can handle exceptions using the try/except statement.
- It explains the use of try-except-else to control the following of a program in the event of an exception.
9. Python Loop:
- You must be able to understand what the statement otherwise means.
- You need to know the entire statement.
10. Python Functions:
- It shows how to repackage a quadruple so that the elements can be divided into multiple variables.
- Learn how to define partial functions.
- This document shows how to type hint parameters.
Essentials Python Learning Strategies
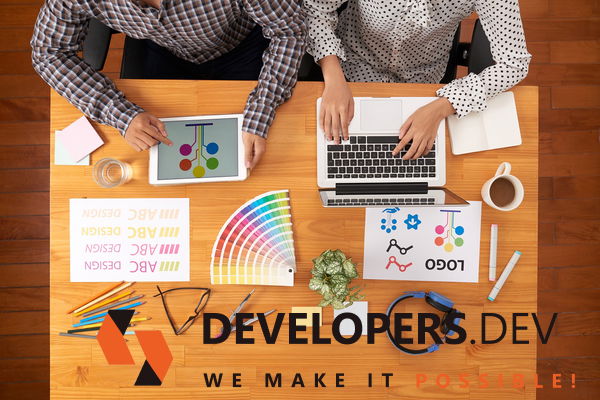
Below are the essential Python Fastest Learning Strategies:
- The basics of Python are essential.
- Set a study goal.
- Choose the best Python resources, including interactive websites, videos and other non-interactive resources.
- Consider learning Python Library.
- Anaconda can help you speed up your Python installation.
- Choose and install an IDE.
- Google can be used to help you troubleshoot your code.
What are the Different Ways of Coding Python?
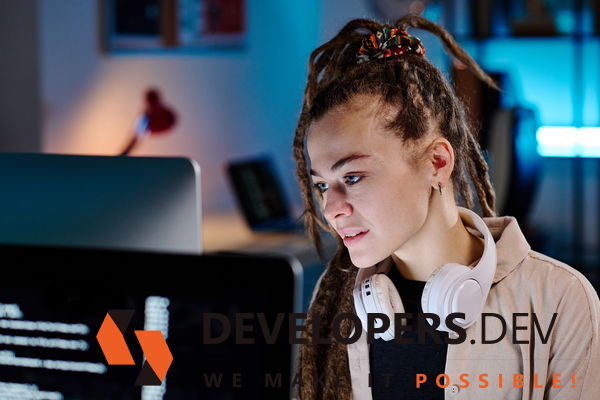
- Python interpreter the Shell is a language. The code is executed line-by-line. Python offers a Python Shell that executes one Python statement and shows the results. Open the Terminal window or the Command Prompt on Windows to run the Python Shell. Enter Python after you have written it. Python prompt is composed of three greater-than symbols.
- IDLE python includes an integrated learning and development environment, often abbreviated to IDLE. This is a group of tools that helps you to write code faster. While there are many IDEs to choose from, Python IDLE is the best for beginners.
- It is easy to use as a text editor with code formatting and syntax highlighting. Code editors are capable of executing code and controlling a debugger.
What is Required for a Good Python Coding Environment?
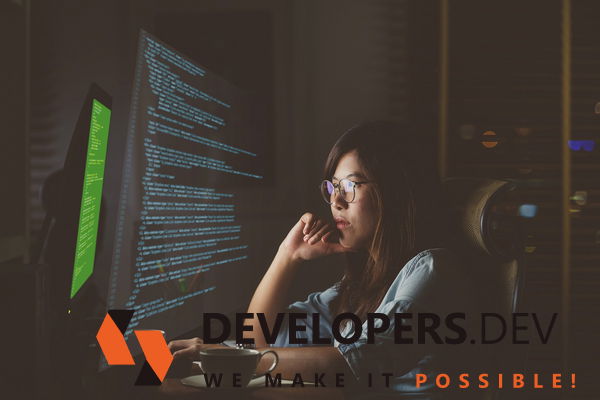
The following are some essential requirements for a successful Python programming environment:
- A good code editor should be included in the environment. Code editors are text editors with special functions for editing and writing code. Atom, Sublime Text and Visual Studio Code are some of Python's most popular code editors.
- The Python environment is only complete with the Python interpreter. It is a Python program. The Python interpreter can run interactive code or code that is stored in a file.
- Libraries are a must in any environment. Libraries are code collections that extend Python's functionality. NumPy and SciPy are some of the shared libraries that Python has.
- Debugging tools should be available in the environment. The purpose of debugging tools is to find and correct errors within code.
- Version control is a must in the Python environment. Version control helps you manage changes in code. Popular version control systems are Git and Subversion.
Tool programs assist in development activities such as testing and debugging. Python's standard tools include the Python debugger and package manager.
Want More Information About Our Services? Talk to Our Consultants!
Experience Level and End Goal Determines the Bifurcation of a Group
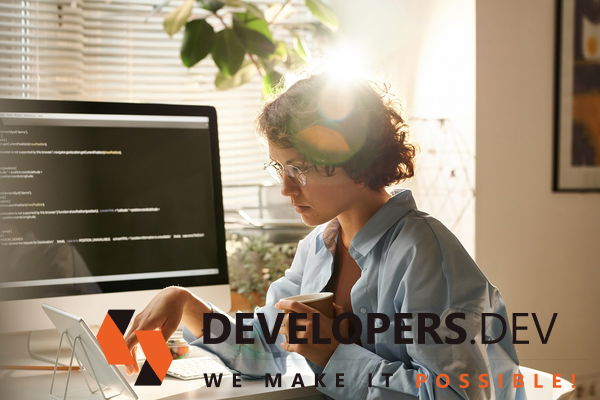
Python IDEs are divided into two categories: those for advanced developers and those for novices:
- Experienced developers often choose IDEs with more features that allow them to personalize the environment according to their needs.
- Beginners often choose IDEs that have fewer features and are simpler to use.
- PyCharm is the most popular IDE for Python programmers. PyCharm offers some features, including code completion and navigation, as well as refactoring and debugging. Visual Studio Code, a lighter IDE popularized for its extensibility and ease of use, is a lightweight IDE.
- IDLE is a very simple IDE that comes with the Python standard library. On the other hand, Pythonista has a more full-featured IDE consisting of a code editor and debugger. IDLE, a simple IDE, is included in the Python standard libraries. Pythonista, a full-featured IDE, includes a debugger and an interactive shell.
Android App with Python
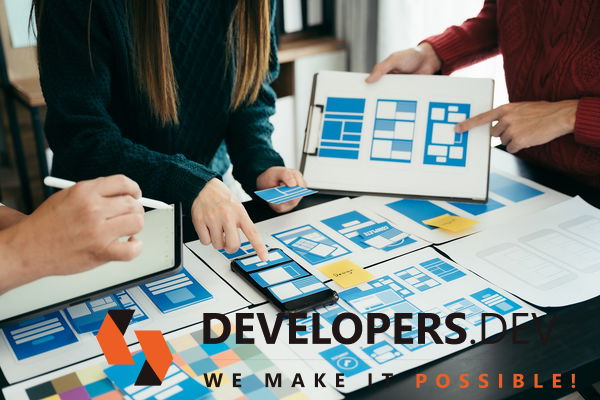
Developers will require platforms compatible with Python code since Android apps will run on Android Operating System.
Developers should be aware that while Android can run Python-based apps, Java remains the preferred language for Android.
Suppose developers want to create Android apps using Python. In that case, they'll need to use a "third-party" tool which acts as an adapter to make Python run natively on mobile devices.
Today, there are many tools available on the market to assist developers.
Python Tools For App Development
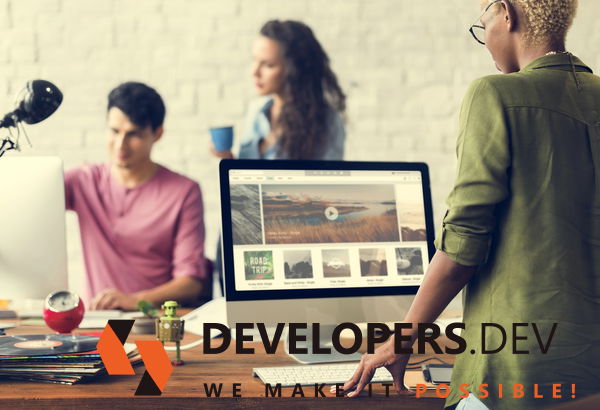
The versatility of Python is a key factor in today's industry. Developers use Python to create all kinds of apps, including Blockchain and command-line applications, games, business and web apps.
We have yet to begin to list all the specialized applications like image processors and web crawlers. Let us answer your question: What keeps you from creating popular apps? This section will cover some Python tools to help you develop these applications.
1. Kivy
The popular framework Kivy allows Python programmers to build innovative mobile applications across multiple platforms, such as Android, iOS and Linux.
Kivys best feature is that it has a Natural User Interface. Let me explain what NUI is. The users will intuitively discover hidden features and interactions offered over time.
The user interfaces on our phones and tablets are very similar.
Kivy is a powerful tool equipped with all the tools a developer could need to build a beautiful app. This includes support for a variety of native inputs.
The OpenGL ES 2 graphics library powers Kivy. Kivy also has a large collection of widgets and customizable support.
Kivy has documentation that can help you with your Android project.
This documentation includes a guide that shows you how to create your Android APK.
2. QPython
With each update to their operating system and silicon, modern Android devices will become a powerful beast. We want to use this power to run Python scripts and programs on our phones and tablets.
QPythons best-known feature is its script engine. QPythons script engine for devices has an interpreter console editor and an SL4A SL4A Library that allow developers to run Python code directly on their devices.
QPython has an integrated environment with a Bottle library to develop WebApps and SL4A for Android API calls. QPython can run a wide range of apps, including WebApps, GUI-based applications, backend apps and more.
There is limited support available for libraries. There are only a handful of precompiled libraries, including Kivy and NumPy.
3. PySide
The PySide2 Module, developed by Qt's team and formally called the Qt For Python Project, provides Python bindings to the Qt App Development Framework.
PySide2 provides Python developers with an array of Qt tools that allow them to create amazing GUIs and develop entire apps.
Three main modules, listed below, drive PySide2s core. However, the ability to add additional modules exists.
Qt Core is a non-GUI version that offers features such as base classes for item models, serialization and signal slots.
Qt GUI Qt cores functionality is extended with GUI-specific features, such as OpenGL, screens and windows, 2D images and paintings, and rasterized 2D painting.
The Qt widgets are a vast array of visual elements and interactive tools that you can use. PySide2 is the new version of PySide that allows you to work with Qt6.
This gives you a set of updated tools to make sure Qt works to its maximum potential.
4. BeeWare
BeeWare, a collection of open-source libraries and tools, allows you to create Python apps on multiple platforms, including desktop, mobile and web.
BeeWare allows you to create apps that can run on Android, iOS and Windows. You also have the option of using MacOS or Linux.
BeeWare is powered by a suite of libraries, tools and utilities that includes:
Toga is a widget development toolkit that works on all platforms. Briefcase is a Python package for distributing projects.
Rubicon ObjC allows developers to access Objective C libraries from Python on iOS or macOS. Rubicon Java allows developers to use Java libraries in Python.
Precompiled Python builds for all platforms that do not have official versions.
You can find a detailed guide to using this tool in the BeeWare tutorial on their website.
5. SL4A
The Scripting Layer For Android by Damon Kohler (SL4A), a useful tool, allows Android developers to run and create scripts using a small collection of languages.
You can find the answer to why you should use scripting language in this article by looking at how responsive these scripts are.
You don't need to edit, recompile, or run your code every time. These scripts also have access to Androids APIs like any native application.
This results in an extremely simple program interface.
The tool currently supports scripts created in Python, Perl and Ruby. It also allows users to create scripts using JavaScript, Tcl or Rexx.
The main GitHub branch has yet to be improved. Still, other developers have updated the code to work on Android Lollipop or Marshmallow.
QPython (which we discussed a few seconds ago) also includes a built-in fork.
6. Pyqtdeploy
PyQt is a cross-platform Python binding set for Qt's widely used and tested application framework. Pyqtdeploy, on the other hand, is a tool that allows you to deploy applications created with PyQt.
Using the pyqtdeploy, you can deploy your Python applications to major platforms such as Windows, Linux, macOS, iOS and Android.
The pyqtdeploy tool has three executables: pyqtdeploy -sysroot, pyqtdeploy -build, and pyqtdeploy.
The three executables build the application using the source code and other parts external to the platform. After they are built, the files will be stored in a special directory for each project.
This is defined by a file called sysroot. This file is what references the earlier files that were created for the creation of the target-specific sysroot.
You can read the Project Descriptions section of PyPIs website to learn more about how everything works.
7. Chaquopy
Chaquopy, a Python SDK that is used to develop Android apps and brought to us by Chaquo Ltd. You can build Android apps using a variety of programming languages, including Python, Java and Kotlin.
It will help developers with a great Python application that has yet to target Android. Chaquopy gives developers the option to choose between two different options.
The Java API allows developers to add the Python component to any Java, Kotlin or app. You can also use the Python API to create the Android application in Python.
Chaquopy can be downloaded as an Android Studio plugin. Gradle, Android Studios build system, handles both the installation and download of the plugin.
This requires almost no developer involvement.
8. Termux
Termux has been widely used for over a decade by Android enthusiasts interested in software application development companies.
Termux is a Linux-based terminal that allows developers to access the Android OS. Termux gives you access to some Linux features, including:
SSH provides secure access to your servers. There are many Linux shells available, including Bash, Zsh and Fish.
There are many text editors available, including Vim and Emacs. The languages of Python, Perl, Node.js and Ruby.
The APT command offers some packages. Support external keyboard and mouse to improve productivity.
Termux allows Python programmers to not only run their applications on smartphones but also to create them. The app is available from different sources such as Google Play Store F-Droid, and Nethunter Store.
Read More: The Programming Structure of Python
What Are the Pros and Cons of Developing Android Apps With Python?
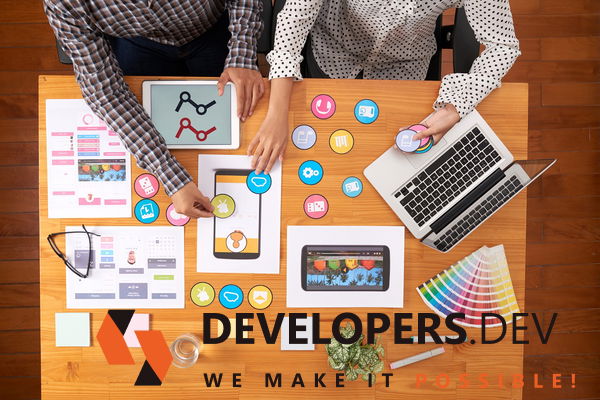
The Pros and Cons of Using Pros
Python is one of the top coding languages. The popularity of Python has many reasons, which we will list below:
Check out the latest news: Private jet and on-demand flight booking apps to be launched in 2023. Python is one of the popular programming languages.
The Python framework is a great tool for developing Android apps.
1. Faster Programming Performance
Python does not need a compiler, as the interpreter is the only one that will execute the program. Python is a great language because it takes less time to execute code, and it's easier to catch errors.
2. Compatibility with Test-Driven Development
Python simplifies prototyping for application development. The language fully supports prototyping and, through the refactoring of prototypes, allows you to build applications from them.
Python can be used to run and develop code on multiple platforms.
3. Variety of Libraries
Python is a powerful tool for developing Android apps. You can choose modules that meet your needs from a wide range of robust and effective libraries.
4. Strong Community
Developers and near coders play a vital role in developing any programming language.
Python has a vibrant community that shares countless guides, tips, tutorials, and useful documentation. Python has a distinct competitive advantage.
You Can Also Find Out More About the Cons
Google has declared that Java is Android's official language. Java is the official language of Android. Other languages we use might need to measure up.
Although Android application development with Python is possible, it will not be as effective as Android apps developed using Java.
Here are some of the possible problems:
- The frameworks may not be able to support all features of Android.
- Our tools can only be used sometimes.
- The native compilers may not be able to optimize the code as quickly because they first generate native code.
- High-level graphics and 2D or 3D graphic support may sometimes be unavailable.
The Best Android IDE in 2023
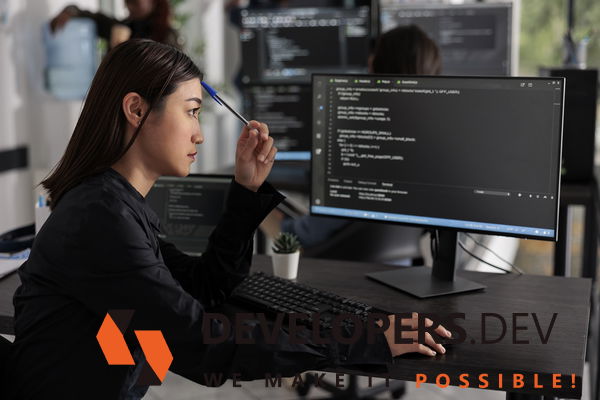
Android Studio
Google created Android Studio as a set of tools to assist developers in creating high-quality Android apps. The suite of tools allows developers to create apps quickly.
The IDEs have been designed for beginners. This IDE has high-level capabilities to make it easier for you to develop Android applications.
This is an emulator that's fast and packed with features. It also offers advanced features like code and project templates, which allow you to check out the navigation drawer and viewer page quickly.
IntelliJ IDEA
Developers use it to develop apps for Android. Intelligent IDEA has been rated the most effective tool for creating Java apps for Android.
This powerful tool allows programmers to develop stable, fast, responsive applications. The brilliant code editor will allow you to create Android applications using Kotlin.
Java, Groovy and Scala. The IDE aims to increase productivity through a highly intuitive code assistant and replace programming languages such as Python, SQL and PHP.
Eclipse
Eclipse, a free and open-source tool that can be used to develop applications compatible with different programming languages, is great.
The tool is widely used and has many configurations. The Java language is the primary programming language used to develop applications.
The software supports C++ and PHP, as well as other programming languages.
Eclipse is the ideal tool to use for Android Development if you are working on large projects and need more customization.
Want More Information About Our Services? Talk to Our Consultants!
Conclusion
We recommend that every developer use these tools as they can help reduce the time it takes to get your app to market while also making maintenance easier and ensuring cross-platform compatibility.
What do you think? What are your thoughts? After you understand these Python tools well, we recommend that you read one of my previous articles, where we discuss a few fun and challenging projects for offshore Python developers.