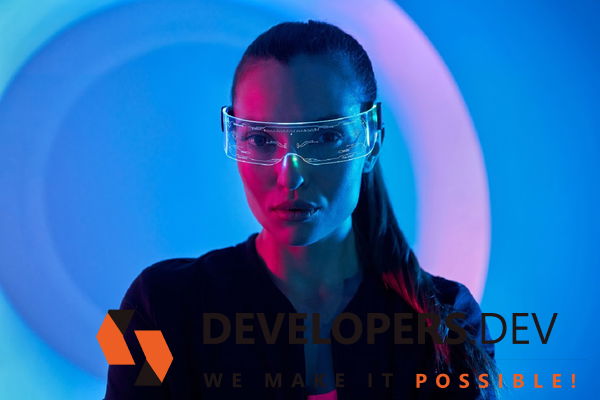
In today's fast-paced tech world, the demand for smarter and faster software is growing rapidly. This makes efficient application development more important than ever before.
But with big projects come big challenges. Developers need better ways to solve problems, especially when time and resources are tight.
That's where dynamic programming (DP) comes in. It's a simple but powerful way to solve hard problems by breaking them into smaller parts and reusing answers instead of starting from scratch each time.
DP helps make software faster, more efficient, and easier to maintain. It's used everywhere, from coding interviews to real-world apps that run in the background of our daily lives.
Compared to brute-force methods or plain recursion, DP saves time by cutting out repeat work.
What Is Dynamic Programming in Simple Terms?
Dynamic Programming, or DP, is a method used to solve complex problems by breaking them into smaller parts. It solves each part only once and saves the answer.
If the same part comes up again, it just uses the saved result. This makes the process faster and more efficient.
The idea behind DP was introduced in the 1950s by Richard Bellman, a mathematician who worked on decision-making problems.
He created DP to make solving large problems easier by reusing solutions to smaller ones.
Let's look at a simple example. Say you want to find the shortest way to climb a staircase, and you can take one or two steps at a time.
Instead of trying every single path, DP stores the best solution for each step. Then it builds the answer from those saved results.
Now, not every problem can use DP. For DP to work, two key rules must apply:
- Overlapping subproblems
This means the problem has smaller parts that repeat. DP stores the result the first time, so it doesn't need to solve the same part again.
- Optimal substructure
This means solving the smaller parts in the best way helps solve the whole problem in the best way. If the smaller answers help build the final answer, DP will work.
Now, here's how DP is different from other methods:
- Recursion solves problems by calling itself again and again, but it often repeats work. DP avoids that by remembering results.
- Divide and conquer breaks problems into smaller ones, solves each once, and combines them. It doesn't reuse answers like DP does.
- Greedy algorithms make the best choice at every step. But they don't always give the best overall solution, especially for complex problems.
Knowing the difference helps you pick the best tool for your coding problem. Use DP when parts repeat, and you can build the full answer from smaller ones.
What are The Core Concepts in Dynamic Programming
Dynamic Programming (DP) is a method for solving complex problems by breaking them into smaller, easier parts. If you're new to algorithm design and wondering what is dynamic programming, think of it as a smarter way of solving problems by remembering solutions to subproblems to avoid doing the same work repeatedly.
To use DP effectively, you need to understand two main approaches:
Top-Down Approach (Memoization)
The top-down approach starts with the main problem and breaks it down into smaller subproblems. Each time a subproblem is solved, the result is saved in a storage area called a memo.
This way, if the same subproblem appears again, the stored result is used instead of solving it again.
Think of it like asking for help from a friend every time you get stuck. Once your friend solves a step, you remember the answer and don't ask again.
For example, in the Fibonacci sequence, calculating fib(5) requires fib(4) and fib(3). Instead of recalculating fib(3) multiple times, memoization stores the value after the first calculation and reuses it.
Bottom-Up Approach (Tabulation)
The bottom-up approach solves the smallest subproblems first, then uses those solutions to build up to the final answer.
Unlike the top-down approach, this method does not use recursion. Instead, it fills a table or list step-by-step, solving from the base cases upward.
Imagine climbing stairs one step at a time. You first figure out how to get to the first step, then the second, and so on until you reach the top.
Each step depends on the answers to the steps before it.
For example, to find fib(5), the program first calculates fib(0), fib(1), then fib(2), building on each result until it reaches fib(5).
This stair-step logic is at the heart of understanding what is dynamic programming.
Defining States and Transitions
The heart of any DP solution lies in clearly defining states and transitions.
- State: A state represents a specific condition or subproblem in the solution process. For instance, in the Fibonacci problem, a state is the position n in the sequence.
- Transition: A transition is the rule that tells you how to get from one state to another. It shows how smaller problems connect to form bigger ones. For Fibonacci, the transition is fib(n) = fib(n-1) + fib(n-2).
When tackling a DP problem, first identify what your state represents. Next, determine how to move between states using transitions.
These steps are essential to mastering what is dynamic programming in both theory and application.
Time and Space Complexity
Dynamic Programming is powerful because it saves time compared to simple recursive solutions. However, this comes with a cost in space, since DP stores results for reuse.
- Time complexity improves drastically with DP. For example, the naive recursive Fibonacci calculation takes exponential time (O(2ⁿ)), while both top-down and bottom-up DP solutions take linear time (O(n)).
- Space complexity depends on how much memory you use to store subproblem solutions. Top-down approaches use extra memory for the call stack and memo storage. Bottom-up solutions use memory to keep the table or array.
Understanding these trade-offs gives a better answer to what is dynamic programming in practice.
When and Why to Use Dynamic Programming
Dynamic Programming is best when:
- A problem can be divided into smaller, repeating parts (overlapping subproblems).
- The solution to the whole problem depends on the solutions to its smaller parts (optimal substructure).
Here are some practical situations where DP shines:
- Route Planning: Finding the shortest or cheapest path in maps or GPS systems.
- Resource Management: Budgeting or scheduling tasks with limited resources.
- Machine Learning: Calculating probabilities or building models that depend on past computations.
- Coding Interviews: Many common problems, like knapsack, coin change, or longest common subsequence, rely on DP.
If you're wondering what is dynamic programming good for, these use cases offer a clear answer.
Read More: Best Programming Languages in 2025
Popular Dynamic Programming Examples
Dynamic programming examples help you see how this method solves real problems faster and smarter.
Let's look at some classic problems with simple explanations.
Fibonacci Sequence
The Fibonacci sequence is a common example to understand what is dynamic programming. The goal is to find the nth number in the sequence where each number is the sum of the two before it.
- Brute-force: This method recalculates many values multiple times, making it very slow.
- Memoization: Stores results of subproblems to avoid repeating calculations.
- Tabulation: Builds the solution from the bottom up, storing answers in a table.
Each method shows how dynamic programming improves efficiency.
0/1 Knapsack Problem
Imagine you're packing a backpack with items, each having weight and value. You want to maximize the total value without exceeding the weight limit.
This is the 0/1 Knapsack problem.
Dynamic programming helps by checking each item and deciding whether to include it. It builds up a table showing the best value possible for each weight limit.
Longest Common Subsequence (LCS)
LCS finds the longest sequence that appears in the same order in two strings. It's widely used in bioinformatics for DNA comparison and in text processing to find similarities.
Dynamic programming breaks the problem into smaller parts and builds a matrix to track common subsequences.
Coin Change Problem
This problem asks: Given coins of different values, how do you make change for a specific amount using the fewest coins?
Dynamic programming tries all combinations efficiently by storing results for smaller amounts.
Edit Distance (Levenshtein Distance)
Edit distance measures how many changes it takes to turn one word into another. It's important in spell checkers and natural language processing (NLP).
Dynamic programming builds a grid to compare characters and count edits like insertions, deletions, or substitutions.
If someone asks you what is dynamic programming, these classic examples illustrate the concept effectively.
Applications of Dynamic Programming in Real Life
Dynamic programming is a powerful technique that solves complex problems by breaking them into simpler parts. Its flexibility makes it useful across many fields.
Here's how the applications of dynamic programming show up in different industries:
Software Engineering
In software engineering, dynamic programming helps make code faster and more efficient. It reduces repeated work by saving previous results.
This improves how programs run and respond.
-
Code Optimization:
- Avoids repeating calculations by storing results.
- Speeds up algorithms like sorting and searching.
-
Caching Mechanisms:
- Saves results of expensive function calls.
- Reduces server load and response time in web apps.
Finance
Finance uses dynamic programming to make smarter investment choices. It helps analyze many scenarios quickly to balance risk and return, making money management more reliable.
-
Portfolio Risk Management:
- Balances returns by evaluating multiple investment scenarios.
- Helps minimize losses while maximizing gains.
-
Optimization of Asset Allocation:
- Allocates resources efficiently based on market conditions.
- Supports long-term financial planning.
Artificial Intelligence and Machine Learning
Dynamic programming is a key part of AI and machine learning.
It helps machines learn the best decisions by evaluating past actions and their rewards.
-
Reinforcement Learning:
- Helps machines learn optimal strategies through rewards.
- Used in robotics, gaming, and autonomous systems.
-
Markov Decision Processes (MDPs):
- Models decision-making in uncertain environments.
- Finds the best action sequences using DP techniques.
Operations Research
Operations research applies dynamic programming to manage resources and schedules efficiently. This helps companies save time and costs in day-to-day tasks.
-
Inventory Control:
- Helps decide how much stock to keep at any time.
- Avoids both shortages and overstock situations.
-
Scheduling Tasks:
- Plan manufacturing processes efficiently.
- Organizes delivery routes to reduce fuel and time.
Bioinformatics
Bioinformatics relies on dynamic programming to compare and analyze biological data. It's essential for understanding genetics and developing medical solutions.
-
DNA Sequence Alignment:
- Finds matching regions between DNA strands.
- Aids in identifying genetic similarities and differences.
-
Genetic Analysis:
- Supports research in disease and drug development.
- Tracks evolutionary relationships among species.
Gaming
In gaming, dynamic programming makes artificial intelligence smarter.
It guides characters to make smart moves and find the best paths in complex worlds.
-
Pathfinding Algorithms:
- Helps game characters find the shortest or safest path.
- Implements variations of the A* algorithm for navigation.
-
Strategic Decision Making:
- Enables AI to plan moves in complex game environments.
- Creates challenging gameplay for users.
These examples highlight how dynamic programming solves real-world problems efficiently. Its ability to handle complex decision-making and optimization tasks makes it a crucial skill in many industries today.
especially in areas like AI and machine learning in modern game development.
Dynamic Programming in System Design and Architecture
Dynamic programming isn't just for solving tricky algorithm problems. It also plays a big part in designing real-world systems that need to be fast, efficient, and able to handle large amounts of data.
From web servers to analytics engines, DP helps make smarter decisions in real time.
Below are some key ways dynamic programming fits into system architecture and design.
DP in Caching Strategies
Caching is used to store results so the system doesn't have to compute them again. This saves time and boosts performance.
- Common in web servers, APIs, and databases.
- Works like memoization by keeping the results of past work.
- Tools like Redis or local in-memory caches use this idea to avoid repeating expensive operations.
Stateful Services and Decision Engines
Many services need to remember past actions to make the right decision now. DP helps keep track of these past states and choose the best path forward.
- Useful in recommendation engines that suggest what to watch or buy next.
- Helps real-time bidding platforms make fast and accurate decisions based on user behavior.
Load Balancing and Task Scheduling
When distributing tasks across servers or systems, it's important to choose the most efficient way.
- DP helps by using cost functions to decide where each task should go.
- This reduces delay and improves system speed by spreading out the workload evenly.
Real-Time Analytics Pipelines
In systems that analyze data on the fly, repeating the same work slows things down. DP cuts down on this by reusing earlier results.
- Helps with monitoring dashboards, fraud detection, and trend tracking.
- Makes time-series analysis faster by avoiding duplicate calculations.
Read Also: Static vs.
Dynamic Typing: How It Affects Code Safety and Speed
Common Pitfalls and How to Avoid Them
Dynamic programming can be powerful, but it's easy to get stuck if you overlook a few key ideas. Whether you're new to DP or refining your skills, knowing what not to do can save you hours of debugging and confusion.
Let's walk through some of the most common mistakes and how to avoid them.
Misidentifying Overlapping Subproblems
One major reason DP fails is that the problem doesn't have overlapping subproblems.
- Mistake: Trying to use DP when the subproblems don't repeat.
- Fix: Before jumping in, check if the same subproblems appear in your recursive calls. If not, a different strategy might work better.
Poor State Definitions Leading to Wrong Solutions
If you don't define the DP state clearly, the solution might not cover all edge cases or might give wrong results.
- Mistake: Missing part of the input that affects the result, like indexes or remaining weight in knapsack problems.
- Fix: Write down what each state represents. Be sure to include all the variables that can change the outcome.
Redundant Recursive Calls Without Memoization
Recursive solutions without memoization often repeat the same work, making them slow and inefficient.
- Mistake: Writing pure recursion and ignoring the idea of storing results.
- Fix: Use a memoization map or array to store answers for known inputs. This cuts down the time complexity from exponential to linear or polynomial in many cases.
Ignoring Base Cases in DP Formulation
Every DP solution needs solid base cases. If these are wrong or missing, the whole solution might fail.
- Mistake: Forgetting to define when the recursion should stop or return a known value.
- Fix: Start by writing the base cases. Make sure they cover the smallest inputs where you already know the answer.
Inefficient Space Usage in Tabulation Approaches
DP tables can eat up memory, especially in bottom-up solutions. Often, you don't need to store the entire table.
- Mistake: Using full 2D arrays when only the last row or value is needed.
- Fix: Try optimizing space by keeping only what's necessary. Many problems let you reduce 2D tables to 1D arrays with a bit of smart indexing.
Avoiding these pitfalls not only improves your DP solutions but also helps you write cleaner, faster code.
Conclusion
Dynamic programming is one of the most powerful tools in problem-solving. It breaks down complex problems into smaller parts and helps find the best solution efficiently.
From coding interviews to real-world systems, DP plays a key role in building smart, scalable applications.
If you're just getting started, begin with simple problems like the Fibonacci sequence or the coin change challenge.
These help build the right mindset and teach you how to recognize patterns. Over time, you'll see how DP shows up in many areas, like finance, AI, system design, and bioinformatics.
Whether you're a developer, engineer, or decision-maker, understanding dynamic programming gives you an edge.
It's not just about solving puzzles, it's about designing better solutions across industries.
Frequently Asked Questions (FAQs)
How do I know if a problem can be solved using dynamic programming?
You can often apply dynamic programming when a problem shows two main traits: overlapping subproblems and optimal substructure.
If you notice you're solving the same smaller problems multiple times and can build the final answer from these repeated results, it's a strong case. This fits the dynamic programming definition and makes it easier to spot dynamic programming examples in practice, like Fibonacci sequence or coin change problems.
What is the difference between greedy algorithms and dynamic programming?
Greedy algorithms go for the best immediate choice at each step, which doesn't always lead to the best overall outcome.
Dynamic programming, in contrast, evaluates all possibilities and stores sub-results to avoid repeating work. A classic dynamic programming example is the knapsack problem, where greedy fails but DP gives the correct result by considering combinations.
Can dynamic programming be used in front-end development?
Yes. While dynamic programming is typically seen in backend systems and competitive programming, it can also solve problems on the front end.
You'll find dynamic programming examples in animations, optimizing DOM operations, and UI pathfinding. In single-page applications, it helps cache layouts and speed up rendering logic.
Is dynamic programming used in database query optimization?
Absolutely. Many SQL engines apply DP under the hood to improve performance. It fits the dynamic programming definition by breaking a query into subqueries and reusing computed results.
This approach is crucial in handling large joins or nested queries efficiently.
What programming languages are best for learning dynamic programming?
Python and Java are widely recommended due to their clear syntax and strong community support.
Python is great for beginners, while Java's strict structure is helpful in understanding memory and recursion depth. Either language can help you understand the dynamic programming meaning by working through hands-on problems and studying how sub-solutions lead to optimal answers.
Can I use dynamic programming in distributed systems?
Yes, though it's more complex. In distributed environments, dynamic programming ideas show up in shared caches, service-level memoization, and breaking tasks into smaller, independent sub-tasks.
These systems often require coordination and consistency, making it harder than traditional use cases but still aligned with the core dynamic programming definition.
Ready to Build Smarter, Faster Applications?
Want to solve complex problems and build efficient applications? Developers.dev offers expert dynamic programming support to help you optimize your projects.
Get in touch today and let's create smarter, faster solutions together!