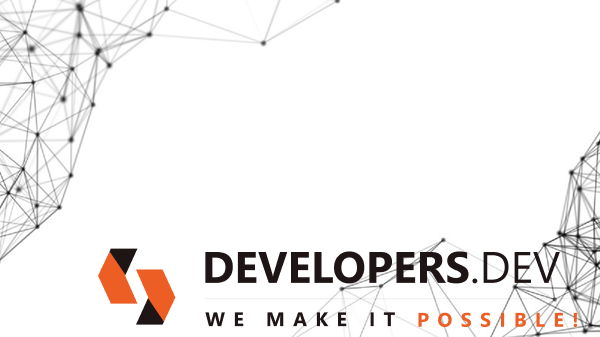
Refactoring old code without altering its exterior behavior is known as Java code refactoring. This procedure seeks to improve both functionality and maintainability while keeping its quality as high as possible - essential elements for effective development, error-free final products, and long-term success.
This article presents various benefits, tips and techniques of effective code refactoring, along with industry best practices and real-world examples. Unleash the power of maintainable, clear code through code refactoring.
Overview Of Java Code Refactoring
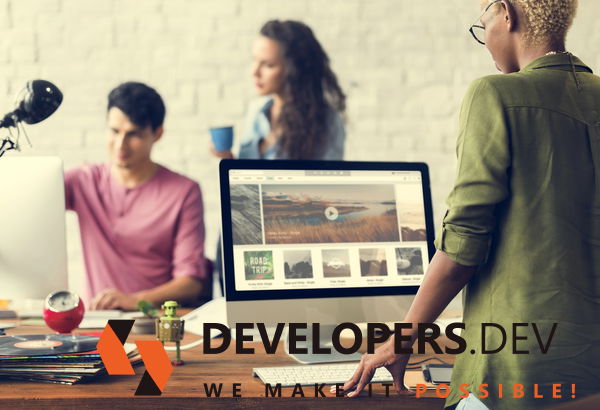
Refactoring Java code involves making small yet incremental modifications to code, including renaming variables or methods, splitting code up into discrete methods and streamlining conditional expressions or switching out composition for inheritance.
Refactoring can help make code more modular and extensible while decreasing duplication as well as getting rid of dead or unnecessary code altogether.
Benefits Of Java Code Refactoring
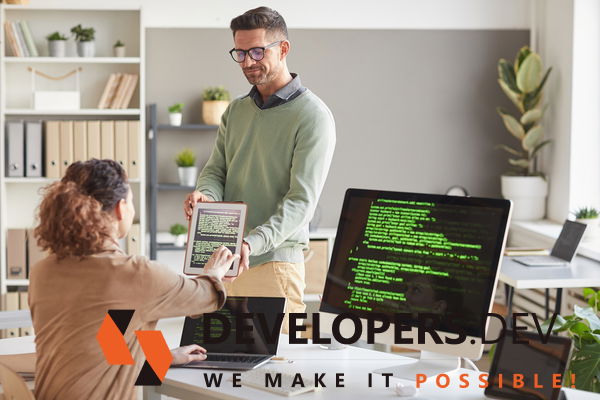
Here we have outlined the important benefits of java code refactoring:
- Improved Readability Of Code: Refactoring can increase the readability of code by giving meaningful names to objects and methods and streamlining code structure further by breaking large, unfocused methods into smaller, manageable methods.
- Simplifying Maintenance: Refactoring makes codebase maintenance much simpler by helping engineers easily locate and solve any potential issues more quickly. Refactoring detects and removes code smells more rapidly so engineers can more quickly identify issues before their code breaks.
- Enhance Code Quality: Refactoring can result in code with higher overall quality by eliminating redundant or dead code, minimizing duplication and improving its structure and design. This approach to software rewriting often produces results in improved code overall quality.
- Refactoring Can Increase Efficiency And Performance: Refactoring can assist in optimizing code by eliminating redundant operations, streamlining algorithms, and using more effective data structures.
- Refactoring Can Improve Reusability: By breaking up functionality into individual classes or methods that may be reused throughout a program, refactoring fosters code reuse.
- Refactoring Facilitates Extensibility: Refactoring makes adding or changing codebase components simpler when needs change, especially code that follows design patterns or concepts such as SOLID, which is more modular, change-adaptive and adapts better over time.
- Promoting Cooperation: Teams working more easily together on an organized codebase are more likely to understand and access it more quickly, leading them to greater cooperation between members.
- Refactoring Helps Decrease Technical Debt: By early identification and resolution of code smells and design flaws that could linger into more difficult fixes later, regular refactoring helps decrease technical debt.
- Refactoring Promotes Consistency: By adhering to industry best practices and adhering to coding standards, refactoring helps maintain consistency within a codebase and makes the developer transition between project components much smoother.
Techniques For Java Code Refactoring
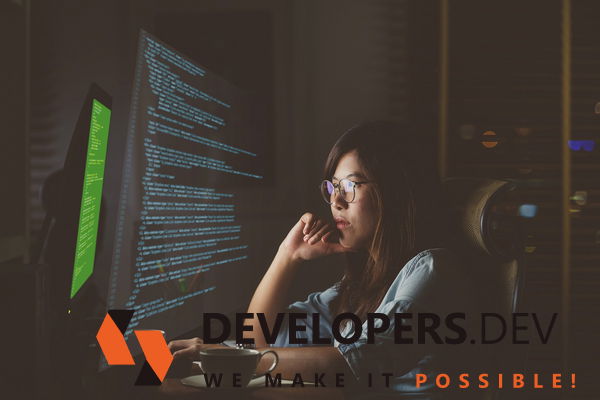
There are other approaches to Refactoring Java code, but two popular ones are the functional and object-oriented (OO) paradigms.
Below we have outlined the techniques for java code refactoring for both OO approach and functional approach:
Refactoring Code Using an OO Approach
OOP (Object Oriented Programming), the practice of organizing code around objects and their interactions, relies heavily on object-oriented methodology (OOM).
This strategy involves encapsulating activity into classes while encouraging code modularity through inheritance, abstraction and polymorphism. Key techniques used for OOM code restructuring in Java include:
- Encapsulate Fields: Make class fields private while public getter/setter methods are made accessible to ensure limited direct access.
- Extract Class: Divide up one large class into several smaller ones responsible for performing specific functions.
- Extract Method: Break down lengthy or complex procedures into smaller, simpler-to-manage steps that each fulfill an independent function.
- Push-Down Or Pull-Up Method: Reduce code duplication while more accurately portraying inheritance structures by moving a method either upwards or downwards through inheritance trees.
- Delegation Should Take The Place Of Inheritance: To encourage composition over inheritance, replace subclasses with distinct classes that assign duties back into their parent classes.
Refactoring Code Using Functional Approach
Functional Programming (FP), at its core, organizes code according to functions and immutable data structures. To allow more modular, expressive code writing using higher-order functions as well as pure functions without side effects - both methods are recommended when applying a functional approach for Java code restructuring:
- Replace loops With The Stream API: Stream API provides a more accessible and condensed syntax than loops while still offering functional approaches to data processing. Parallel processing can take place effortlessly, while actions on collections can include filtering, mapping and reducing.
- Employ Lambda Expressions: These concise ways for creating anonymous functions are increasingly used as alternatives to anonymous inner classes in code development, simplifying syntax while being passed as parameters into functions like reduce, map and filter provided by Stream API functions.
- Unchangeable Data Structures: Immutable or immutable data cannot be changed once created, which increases predictability and simplicity in your code. You can construct immutable structures using Java's final keyword or Collections. Unmodifiable list () function or even Google tools - providing predictability in code as you go forward.
- Extract Function: Extract function involves breaking large, complex functions down into simpler functions that each carry out a singular task - making your code simpler for readers, more reusable, and simpler to test.
- Higher-Order Functions: Higher-order functions refer to functions which return additional functions as their results or accept functions as arguments, making for expressive and versatile customization of functions using Java's Function interface or its various forms like UnaryOperator or BiFunction. These allow for expressive customization options.
Read More: Transform Your Business with Java App Development
Tips For Refactoring Java Code
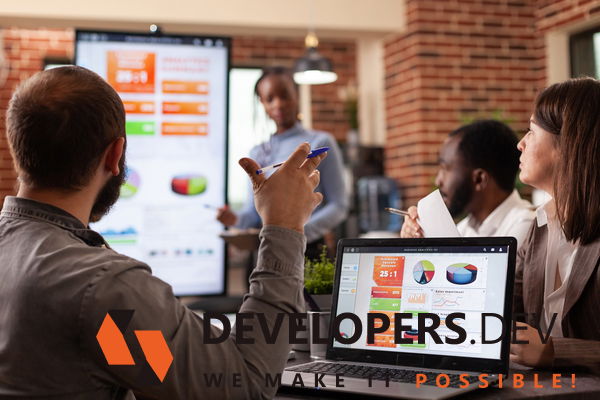
Here we have outlined the important tips for Java Code Refactoring:
- Preserver Functionality: Refactoring Java code with Refactor feature aims at increasing code quality while simultaneously protecting its external behavior, such as how it responds to inputs, outputs and user interactions. Never fear breaking anything when working, it informs you which services depend upon what code you're working on, which require testing, and which may break with modifications.
- Know Your Code Inside And Out: Before undertaking any refactoring efforts, ensure you fully comprehend all aspects of the code you intend to modify - its dependencies, relationships and functional aspects. Doing this will guide your choices while helping prevent changes that would compromise the functionality of the project.
- Divide Your Java Refactoring Procedure Into Steps: Refactoring an extensive software program may seem intimidating at first, but by breaking up the refactoring procedure into more manageable steps, you can minimize chances of mistakes while lightening effort requirements and quickly and reliably validating changes made during refactoring.
- Refactoring involves making changes to your codebase: So they might not go as expected. To save both time and money when updates break your software without knowing why they did so, it would be smart to have backup copies stored separately in different branches.
- Regularly Run Tests On Changes: Refactoring code should never unintentionally cause problems or compromise program functionality, so having tests in place offers an extra safety net to protect the functionality that's already present and ensure your code works as intended. You can use filtering or sorting by "impact" metric in Asset View to better assess what areas might require the most reworking and benefit most from being refactored.
- Use Refactoring Tools: Reworking Java code doesn't have to be challenging when using Eclipse and IntelliJ as your IDEs of choice, with their powerful set of refactoring features, including safe deletes, extract methods/parameters/fields/variables from inline refactoring operations and copy/move capabilities available within IntelliJ. IntelliJ IDEA boasts such tools which simplify your work while decreasing any chance of creating issues during refactoring processes.
- Use Unit Tests: Refactoring should provide developers with peace of mind that their code changes won't cause unexpected issues or break your program by helping identify regressions, verify functionality is working as designed, promote teamwork and ensure long-term maintainability using unit tests. A comprehensive suite of unit tests may prove essential here.
- Utilize Continuous Feedback To Assess Changes In Performance: Refactoring Java code enhances both its structure and performance, so tracking performance metrics provides clarity as to whether or not refactoring efforts have an effect. Tracking performance gains is essential to measuring progress and comparing different Java refactoring techniques. With evidence-based feedback features, you'll always have access to tangible support as you optimize and enhance your codebase. Not every problem needs to interrupt production before being addressed by experts in observability.
- Review Refactored Java Code With Peers: It can often be advantageous to get another developer's perspective once restructuring modifications have been completed in order to gain another viewpoint and point out any flaws you missed or provide fresh perspectives and insightful criticism. Peer reviews also serve to enhance communication among team members as they provide a useful method to promote collaboration among them.
- Record Refactoring Adjustments: Working together with other developers means their work may be affected by your changes, so documenting any rationale behind changes, even when they may seem counterintuitive, is crucial to encouraging cooperation and increasing transparency - this also facilitates onboarding and information-sharing processes more smoothly.
- Regression Testing: Before altering any Java code, make sure that existing functions continue working as intended after any alterations are made. Regression testing allows you to ensure that newly added logic does not conflict with existing logic in any way.
- Team Informed: When working together on a project with other developers, inform them about any refactoring modifications you make so as to prevent disputes and aid their adaptation to any modifications you introduce. Doing this helps both you and them adjust more smoothly when any modifications come about.
Conclusion
Refactoring is a key process that ensures long-term software project success. Any complex or disorganized codebase can be transformed into something readable, maintainable and extendable by applying one of the methods outlined as part of a Java development services with best practices in mind - but keep in mind that refactoring Java code shouldn't just be seen as a one-off event.
Refactoring can become part of daily development cycle activities rather than simply an isolated event.