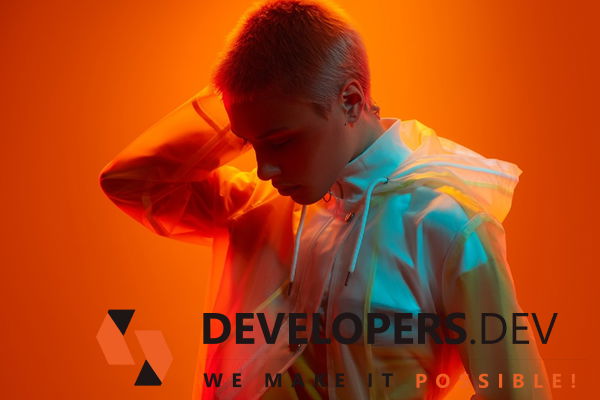
Java exception handling can be an intricate topic; even experienced engineers can spend hours debating which exceptions should be thrown or handled, making the subject harder for beginners.
Due to this complexity, many development teams adopt usage guidelines tailored specifically for them.
Java exception handling is one of the best strategies available to tackle runtime issues caused by exceptions and is integral to software creation.
In this blog, you will learn Java exceptions, their handling methods, hierarchy structure, and various types of exceptions available, along with the best practices that apply.
Defining Exceptions
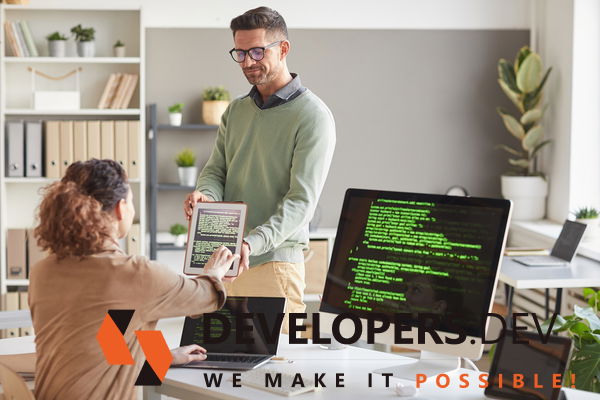
So, what exactly are exceptions? Simply stated, exceptions refer to irregular situations during program execution that fall outside normal expectations.
An exception occurs whenever something goes awry - when files don't exist or when calling methods with null arguments on an object whose variable was null; any instance in which something goes wrong. We call such processes exception handling.
Exception handling is an error-handling mechanism. When something goes wrong in an application, an exception is raised, which can lead to its crash.
Alternatively, you can choose to handle the Exception instead, acknowledging its existence while keeping it from doing so and taking measures necessary for its recovery or graceful failure.
What Does Java's Exception Handling Mean?
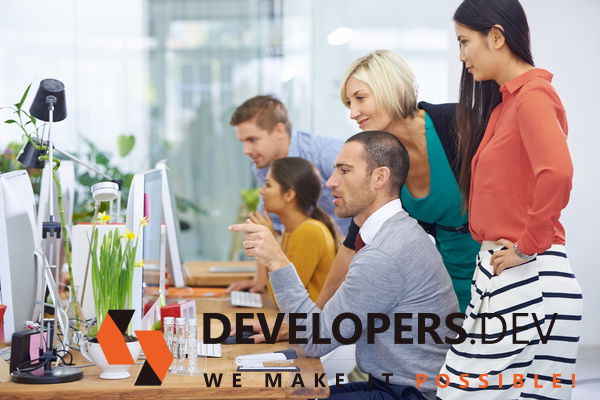
Exception handling in Java provides a way of mitigating exceptions and recovering from them, providing powerful mechanisms that handle runtime exceptions without bugging out programs.
Furthermore, exception handling helps maintain program flow; an exception is defined as any abnormal condition occurring at runtime that disrupts the normal flow of execution.
Exception Handling in Java is an innovative approach to enhancing the convenience and performance of applications written with this programming language.
If mishandled, exceptions could present serious threats that impede their functioning in real time.
Java Exception Hierarchy
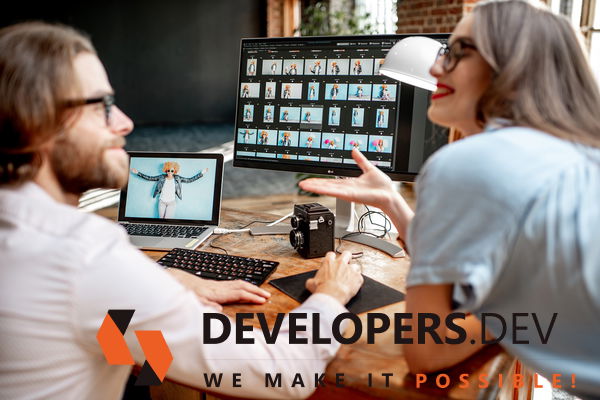
Here is the Java Exception Hierarchy.
-
Throwable: It is the root class in Java for its exception hierarchy and resides within its package java.
Lang.
- Error: a subclass of Throwable; errors are abnormal conditions that cannot be managed or predicted and depend solely on environmental influences. They cannot be addressed and always result in a program interruption, such as when an infinite loop or recursion occurs, such as StackOverFlowError.
- Exception: It represents abnormal conditions that must be handled explicitly if our code continues running smoothly. If handled, handling can ensure continued execution without interruptions to its workflow.
Types Of Exception In Java

Java supports both checked and unchecked exceptions, both of which can be used similarly. Discussions regarding when or whether to utilize each type are more than welcome.
For now, though, let's follow the Java Tutorial approach as set forth therein. Here read out types of exceptions in java:
Checked Exceptions
It's best practice to utilize checked exceptions whenever there are unexpected events you anticipate that your application should be prepared to handle.
A checked exception extends the Exception class; any method throwing or calling one that specifies one should either specify or handle its impact accordingly.
- Checked exceptions at compile time include exceptions that have been verified as checked exceptions.
- These classes extend Exception, except RuntimeException.
- Without proper handling of these errors, your program won't compile.
- Examples of Error Codes IOException, ClassNotFoundException, etc.
Unchecked Exceptions
Unchecked Exception is an extension to RuntimeException that should be used when dealing with unexpected internal errors that you cannot anticipate and which cannot be recovered from by your application.
Methods do not need to handle or specify unchecked exceptions explicitly - typical examples that throw unchecked exceptions include:
NullPointerException or nullPtrException occurs due to missing initialization of a variable, which results in its nullPointerException being raised.
- An IllegalArgumentException may occur from improper usage of APIs, which causes this error message.
- At runtime, exceptions that are being checked could include unchecked exceptions that go undetected.
- RuntimeException subclasses provide their parents a way out.
- Errors occur if not addressed explicitly during runtime.
- Examples of ArithmeticException, NullPointerException, etc.
Advantages Of Exception Handling In Java
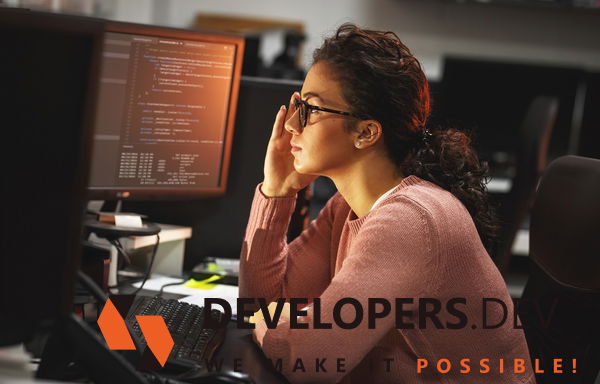
- Separating Error Handling Code From Regular Code
- Error Propagation Up the Call Stack
- Grouping and Distinguishing Error Types
Disadvantages Of Exception Handling In Java
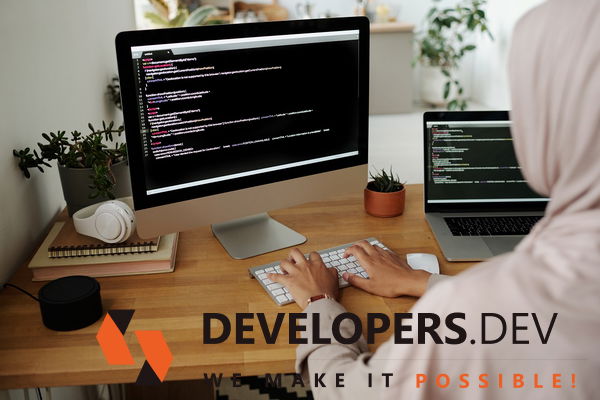
- Are You Facing Unnecessary Expenses?
- Not knowing the inner workings of an application.
- Accumulating logs with active events.
- Unable to focus on what matters in life.
Read More: Transform Your Business with Java App Development
Java Exception Handling Best Practices
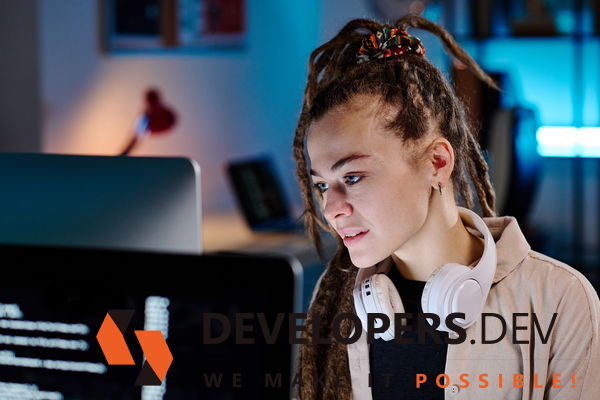
Here read out some best practices for java exception handling:
Clean Up Resources In An Exact Block Or Use An Is-Not-Able Statement
Sometimes, when using resources in a try block like an InputStream that need to be closed afterward, one common misstep is closing them at the end of the try block instead of at its conclusion.
The problem with this approach is that, so long as no exception occurs, all statements within a try block will get executed successfully, and then the resource is closed off automatically; however, you put there a try block specifically because something unexpected could go wrong.
Call one or more methods that might throw an exception, or you generate one yourself; that means the try block may not reach completion, and resources won't get closed off as intended; therefore, it would be prudent to put all clean-up code within either finally block or using try-with-resource statements for efficiency.
Utilize a Finally Block by contrast, unlike your try block's last few lines, which only get executed upon successful execution or handling an exception in the catch block, finally, blocks are always executed.
This guarantees you can clean up all resources opened during the execution of try or catch blocks. In doing this, you ensure all resources you've allocated have been released back onto the server when desired.
Seek Out Specific Exceptions
Be careful that any exception you throw is as specific as possible. Remember that someone unfamiliar with your code (perhaps yourself in several months) could need to call your method and handle its Exception.
By providing them with as much detail as possible, your API becomes more accessible for people to comprehend.
As such, your method's caller can better manage or avoid such instances with additional checks. Therefore, try to identify an event-specific class to throw, such as NumberFormatException instead of IllegalArgumentException, and avoid non-specific exceptions altogether.
Document Any Exceptions That May Apply
Once an exception is included in a method signature, its documentation in Javadoc must also include it. This has the same goal as previous best practices: giving callers as much information as possible so they can avoid or manage an exception.
Add a throws declaration in your Javadoc and describe any situations that might trigger exceptions.
Create Error Exceptions With Descriptive Messages
This best practice builds off of two others by not providing your method callers with any information directly. Everyone reading an exception message needs to comprehend exactly what happened when an exception was reported in their log file or monitoring tool.
Therefore, your problem statement should provide as much pertinent information as possible to grasp an extraordinary event fully.
No doubt; don't compose an essay-length text explaining why there has been an exception, but in just 1-2 short sentences, you can provide enough detail about its cause.
That will enable your operations team to understand the severity of a given problem and allow for a more straightforward analysis of any service incidents that arise.
Exception classes will typically provide enough detail about what kind of error has arisen, allowing you to quickly solve it without giving much additional information.
Locate The Most Specific Incident First
Most IDEs make this best practice easier: when trying to catch less specific exceptions first, an unreachable code block is reported as unreachable - although only the first catch block that matches an exception gets executed.
So if you catch an IllegalArgumentException first, the catch block that should handle a more specific NumberFormatException (it being a subclass of IllegalArgumentException) won't ever get executed.
Always attempt to catch exception classes with more particular facets first and add less specific catch blocks at the end.
Don't Catch Throwable
Throwable is the superclass for exceptions and errors; using it as part of a catch clause would only increase its severity.
Use Throwable in your catch clauses, and it will catch all exceptions as well as errors. Errors may be issued by the JVM when there are serious problems that cannot be managed directly through an application.
Typical examples are OutOfMemoryError or StackOverflowError errors; both result from situations beyond our control that cannot be managed within an application's boundaries.
As such, it would be wise not to catch Throwable objects unless you are absolutely certain you can manage an error appropriately and can handle an exception in an exceptional circumstance.
Don't Ignore Exceptions
Have you ever encountered a bug report in which only part of a use case was executed successfully? That could be due to an ignored exception thrown during development that was thought unlikely, with an accompanying catch block that doesn't log or handle this error condition.
Once you encounter this obstacle, you'll probably hear one or more comments such as, "This will never happen." Don't discount exceptions too quickly: be wary if something seems impossible happening before dismissing any "This won't" comments as infallible truths.
No one knows how the code will evolve; someone could unwittingly remove validation that prevents an exceptional event without realizing its ramifications, or the code throwing the Exception could change and start throwing multiple identical exceptions without being prevented by calling code.
You should at least log a message alerting all relevant personnel that an unexpected occurrence has occurred, and someone must inspect this further.
Want More Information About Our Services? Talk to Our Consultants!
Conclusion
Throwing or catching an exception involves many considerations; most have as their goal improving code readability or API usability.
As exceptions serve both error handling and communication functions simultaneously, make sure your coworkers fully understand your Java exception handling best practices and rules so everyone has consistent usage across their teams, explore our Java development services now.