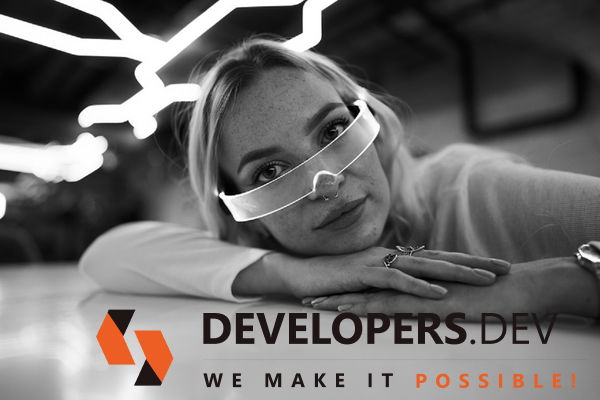
Performance Optimization
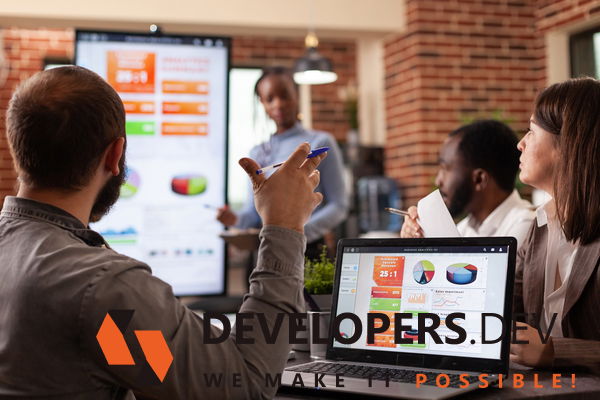
Performance optimization of software and programs refers to the process of improving an application in order to operate more efficiently.
Adopting strategies for tracking and evaluating an applications performance to find ways to enhance it are the keys to an efficient application.
Performance optimization aims at improving one or two aspects of system performance such as execution time, disk space or bandwidth consumption.
To accomplish this goal effectively usually requires sacrifice on one aspect for another - for instance increasing cache sizes can improve runtime performance but increase memory consumption by up to 25 per cent.
Levels Of Optimization
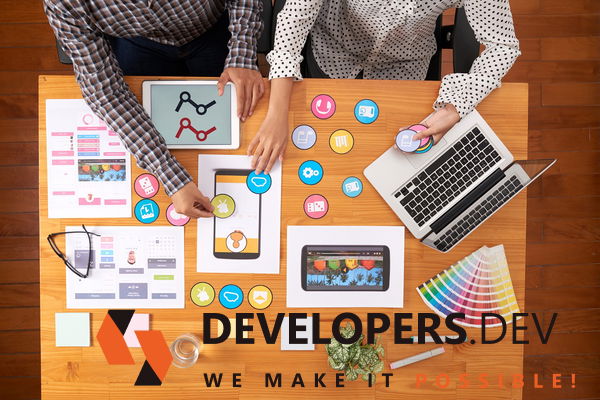
Design Level
- Optimizing a systems design to best utilize available resources depends upon goals and load.
- Architecture of a system is one of the main determinants that shape its performance.
- An optimal strategy to address network latency may involve making less network requests; such an optimization approach would enable systems with high network latency to make less network requests overall.
Data Structures And Algorithms
- Algorithms and data structures play a pivotal role in defining how well any system performs.
- If we want to ensure a system has been optimized, its algorithms must meet certain optimal conditions: constant O(1) time complexity of linear O(n); logarithmic logarithmic log n or log-linear log-linear log n log.
- O(n2) algorithms cannot scale to large data sizes; abstract data types provide better system optimization solutions.
Source Code Level
- Source code selection is also crucial for system optimization when it comes to implementation of algorithms.
Build Level
- It is possible to optimize for specific processors by disabling non-required features of software during the build.
NB Optimizing code or technologies to improve performance can reduce readability, complicate systems, and make them more difficult to maintain and debug.
It is therefore recommended to optimize at the end stage of development.
Client And Server-Side Optimization
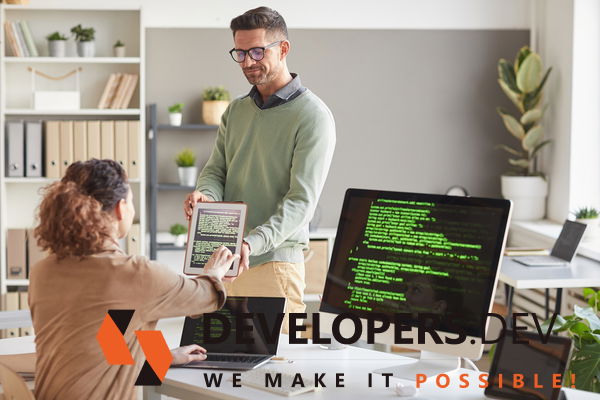
Application code typically comprises client and server-side components that interact together. Both components may experience performance issues.
Client-side performance refers to how results appear within a web browser or user interface, including initial page loading time, resource downloading speed and JavaScript running in the browser as well as image load time etc.
Optimizing server-side performance usually means optimizing database queries and application dependencies in order to enhance its overall efficiency.
Client Side Performance Optimization
Here are some tips to improve performance on the client-side
1.Caching And Content Delivery Networks
- Content delivery networks (CDNs) offer an intelligent solution for handling static files like JavaScript, CSS and image files which do not change frequently.
- Examples of CDNs include CloudFlare (Amazon AWS), MaxCDN, Quickly etc.
- Bundle and Minification
- Performance is improved by bundling files and producing fewer files
- Performance can be improved by removing unnecessary characters, such as white spaces.
- Optimizing image usage
- Images can be made smaller and optimized in most cases
- If you use several small icons, its best to only use Icon fonts. Font awesome.
- Remove duplicate JavaScript and CSS
- The size of the files is reduced by removing duplicate code, resulting in better performance.
- A minimalistic style Framework
- The styling framework should help you with your systems performance as it has been optimized for performance.
Want More Information About Our Services? Talk to Our Consultants!
What Are The Software Development Methodologies?
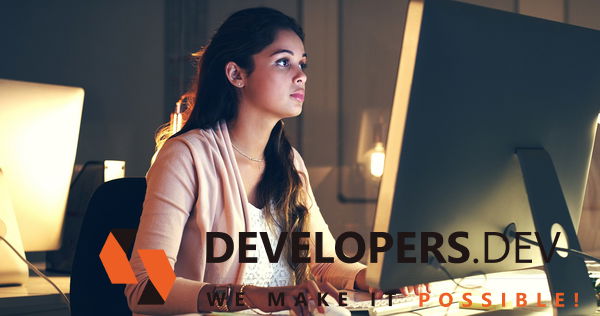
Software Development Methodologies (SDMs) are systematic approaches that aim to plan, structure and regulate the software development process.
SDMs offer guidance and principles designed to guarantee software development projects complete on schedule while staying within their budgetary constraints. Some common SDMs include:
- Agile Methodology
- Waterfall Model
- Scrum Framework
- DevOps
- Lean Development
- Prototype Model
- Rapid Application Development (RAD).
- Spiral Model
- Iterative and Incremental Development
- Extreme Programming
Each methodology offers its own set of advantages and disadvantages; choosing an approach depends on a number of variables including complex project requirements, team composition and size as well as costs/timelines considerations.
Software Development Best Practices
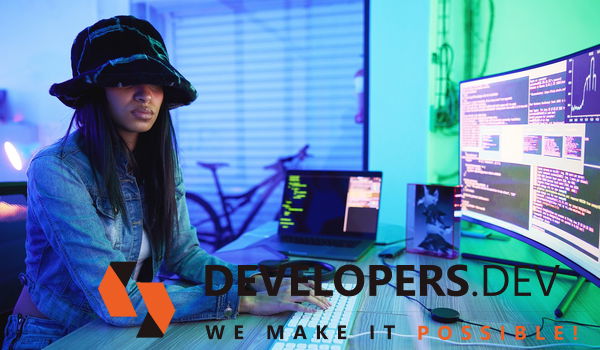
Below are some modern software-development practices that can help you create better software.
1. Clarify The Requirements
One of the key standards and best practices of software development is understanding your products or services requirements, while programmers must implement their programs using specific best practices.
A Software Requirements Specification Document is an in-depth document outlining software requirements and design specs, providing information such as design characteristics, choices, constraints and diagrams so as to prevent further clarification needs.
This will make for more efficient decision making overall and eliminate further clarification needs in future discussions.
One key best practice to follow when working on projects is keeping all projects open for review, so software is rigorously tested before entering its release cycle.
Code reviews, software testing documents and troubleshooting documents should all be utilized; failing this practice could result in bugs that remain undetected and hard to track down.
2. Proper Development Process
Every project must adopt an organized method for developing software. To facilitate this development process, SDLC offers a detailed flowchart outlining specific goals and actions to be taken throughout its development cycle, helping clients and project teams set expectations accordingly.
Depending on project requirements, client needs, specifications and timelines; specific SDLC frameworks may be appropriate depending on individual circumstances.
Agile development works best in fast-paced environments where multiple agile teams collaborate rapidly to identify problems and streamline processes quickly, such as Apple, Microsoft and IBM all use agile development extensively.
The waterfall method of software development is a systematic, carefully considered process used by beginners or new software junior developers in creating programs.
It helps develop their attention to detail as they build programs through this step by step approach to development. While it has fallen out of favor due to sudden changes, those looking for structured approaches such as SDLC (Software Development Lifecycle Center) still benefit from using this methodology.
3. Use An Application Framework
Frameworks provide senior developers with a reusable infrastructure of components and tools designed to streamline development efforts and speed up time-to-market.
Frameworks like these enable programmers to focus on writing code rather than on tedious, time-consuming tasks like file format conversion and database administration.
At the outset of any project it is crucial that an appropriate platform, language and framework be selected because alteration later may prove both time-consuming and costly.
Frameworks can also reduce errors when developing apps by making sure they work on all platforms simultaneously, helping save both time and resources during development of software products.
Read More: What Are the 8 Steps to a Formal Software Development Process In 2022?
4. Make Your Code Easy To Read
Maintaining simple code is always best, not only because this keeps things clearer but also easier for modification and reuse, as well as being the surest way of avoiding an out of control situation.
You can do three simple tests to see if your code has been simplified:
- It is simple to understand and test your code.
- Your code contains logic boundaries which are restricted to one domain.
- The code you have written is shorter than the amount of comments required to explain it.
You can improve the efficiency of coding by keeping in mind these tips and simplifying your code.
5. Working In Pairs Or Integrating Code Reviews
Working in pairs or conducting code review are best practices that software development teams should implement, which will facilitate better communications, collaboration, knowledge transfer, code quality as well as overall team effectiveness.
Your codebase can benefit immensely when reviewed or shared among multiple programmers, while sharing of ideas helps strengthen it even further.
Code reviews can serve to transfer knowledge among members, while encouraging best practices.
Ensuring all team members review each others code can ensure it adheres to a uniform set of standards, making future development simpler.
Integrating code reviews into your development process may bring substantial gains for both team and codebase alike.
6. Write Unit Tests Before You Write Software Code
Continuous testing is key to detecting errors and bugs during software development cycles, so dont wait until all code has been written before beginning testing.
Continuous testing provides you with a deeper insight into what code has been developed and any work remaining that needs to be accomplished.
Unfortunately, pinpointing an individual mistake that leads to bugs can be extremely challenging; hence why incorporating testing into your workflow will become essential.
Before embarking upon continuous testing, one should become conversant in DevOps concepts and shift left testing.
Shift left testing can quickly uncover errors early and reduce disruption from errors that come in later; other strategies for discovering and correcting mistakes include automating regular testing cycles as well as looking into testing as a services model.
7. Use An Issue Tracker To Manage Your Software
Software bug tracking is a means of reporting software bugs during development. With issue-tracking tools in place from day one of a project, developers can identify and address problems earlier and save on expensive fixes down the line.
Therefore, its inclusion should never be underestimated!
8. Use Continuous Integration Best Practices In Software Development
Continuous integration (CI) is an industry standard practice used in software development.
CI involves gathering all changes made by different workers into one repository and then testing whether their changes work together effectively - this enables early identification and resolution of issues, thus decreasing bugs or other complications from appearing later.
Follow these steps to implement best practices for Continuous Integration Software Development:
- Create a shared repository of code, such as Git. All developers who are working on the same project can use this repository to push updates.
- Automate the build process by compiling code and running tests automatically.
- Configure the build to run for every code change. This will ensure that each change has been thoroughly tested.
- Manage the build and test process using a Continuous Integration Server like Jenkins or Travis CI.
- All developers should be able to access the Continuous Integration Server in order to monitor build and test results.
- Set up notifications that alert developers to any failures in a test or build so they can address the issue quickly.
- Assure that all members of the team understand and commit to Continuous Integration.
You can improve your softwares quality by following these best practices. They will help you catch problems early on in the software development process.
This will lead to a successful and efficient software project.
9. Use A Version Control System
A versioning system is one of the best ways for multiple developers to ensure that software will be created correctly.
Version control tools enable developers to work simultaneously on features and bug fixes without risk of overwriting each others codes, without interrupting each others work in any way.
Once errors occur they allow you to go back in and fix any mistakes without disrupting others efforts.
Git is the worlds preferred version control system and its services online as a host for this tool. Their Pull Request feature enhances version control, enabling you to merge redundant and explicit commits together into single commits for improved readability and cleaner commit history management - thus improving Git overall.
Other popular version control solutions are CVS/SVN and Mercurial as alternatives.
10. Sandboxing With A Virtual Machine
Sandboxing is another best practice software developers must abide by. Virtual machines provide a controlled environment in which to test components, upgrade software components, manage dependencies and more.
This allows greater control of sandboxing environments even if running on an image from an untrustworthy third party.
Sandbox restrictions prohibit applications from accessing resources that require special privileges like system calls, files or process management.
Virtual machines provide an extra level of protection for untrusted code by creating an isolated environment within which its execution takes place.
They may use operating systems, application containers such as Docker or even user namespaces of Linux kernel. By doing this, virtual machines help protect an application against attacks which aim to exploit security flaws.
11. Use A Code Signing Certificate
An identity and integrity check of software with a code-signing certificate will help establish your credibility while assuring its quality and longevity.
Coding standards play a pivotal role in software development; using one is even vital.
Signing your software can help protect against unauthorized copies and protect its intellectual property, thus safeguarding its ownership.
With advances in technology, signing has become simpler over time; previously it could have been challenging for software developers who distributed CD-based versions to use a code signing certification to validate them.
Without proper validation procedures in place, any individual could copy an entire software program onto multiple discs without detection.
To address this risk, digital signatures were developed as a reliable method to sign documents and software; users could store software instead of optical media through secure flash drives or similar storage methods; although this did not prevent others from copying or distributing copies.
12. Choose The Right Software
Selecting and employing appropriate software development software is integral to successful software creation and should be tailored specifically for your needs, with features tailored specifically to match them.
When developing mobile apps for example, use software specifically created for such projects.
Finding the appropriate software may seem challenging, but here are a few key points you must remember in order to select it successfully.
Here are a few helpful hints and guidelines designed to make finding software much simpler!
- Determine your needs: Before you look for software, determine what it must do. List the features you need and rank them according to their importance.
- Investigate your options: Investigate the options available that meet your needs. Consider the features, ease-of-use, and compatibility of your existing tools.
- Read reviews.Read the reviews of software that you are considering. You can find reviews of users who are in the same industry as you or have similar needs.
- Try Before You Buy:Many providers of software offer free demos or trials. You can use these to see if the software meets your requirements.
- Cost is always an important factor when selecting software. Consider upfront costs, ongoing maintenance fees, and any license or subscription fees.
- Support: Select software that offers good support including online help, documentation and customer service.
You can improve the software development process and save money by choosing the correct software.
13. Keep Track Of Your Progress
Documenting software development progress is an integral component of best practices in software engineering. Documentation allows you to keep tabs on changes made to code as well as track issues as you work, providing a record that may come in handy later.
Documentation can take many forms: comments, commit messages and project notes. For optimal reading experiences it should be concise yet accessible and organized and kept current so it makes it easy to locate information quickly and efficiently.
Documenting your progress can help prevent repeating past errors. By documenting, you can learn from past missteps and improve future projects.
Plus, documentation allows your team to better comprehend and collaborate on what work has been completed so far.
Documenting your progress can also help you identify patterns and trends. If an issue reoccurs again and again, investigate its source before taking appropriate actions - you could improve work quality while cutting time spent completing future tasks!
14. Style Guides Are Important
Style guides are an integral component of software development. They ensure code remains uniform and maintainable while offering guidelines on writing styles to keep coding easy, clear and comprehensible - saving both time and energy when updating it over time.
Style guides should contain information regarding naming conventions, formatting standards and commenting & coding standards that is easy for developers to understand & utilize.
Furthermore, updates on new standards should occur regularly for best performance.
Style guides help ensure team members comply with uniform code standards. Working as part of a collaborative environment becomes much simpler when team members can read and comprehend each others code easily, helping new team members quickly adjust to project work while decreasing error-prone code production.
An effective style guide can also enhance the quality of your code while decreasing security vulnerabilities.
15. Design Before Coding
To create the highest-quality software applications, it is imperative to follow sound software development principles. Before writing code, it is vitally important to plan and visualize the application thoroughly - creating an in-depth blueprint containing details regarding architecture, functionality, user interface design etc.
Design is key when developing software in order to ensure its users and stakeholders will benefit. Doing this allows developers to identify any potential obstacles early on - saving both time and money later down the line.
Software developers can take advantage of a wide range of design techniques like wireframing and prototyping to develop mockups of software designs before writing any code.
These allow the developers to visualize softwares structure and functionality prior to writing any lines of code.
Before beginning programming, it is also essential that a solid design be undertaken as this can ensure your software can scale and remain maintainable over time.
A thoughtful architecture makes adding future features easier; therefore making maintenance, debugging, and resource savings much simpler and faster than otherwise possible.
16. Do Not Push Too Many Features
One of the key principles in software development is not attempting to include too many features all at once; while this might tempt us, doing so could end up overwhelming users, adding unnecessary complexity, delaying release dates and increasing bugs.
Prioritize features, and add them slowly over time, testing each element and refining before moving onto the next.
This makes code maintenance and debugging simpler while creating an enjoyable user experience.
17. Maintain Production And Staging Environments
Maintaining two distinct environments - staging and production - for software development is highly recommended as this allows developers to test software in an unimpeded setting while debugging issues without impacting users, applying security updates without affecting users, recovering from disasters quickly, performing performance tests efficiently.
Testing in a staging environment can be performed both automatically and manually to make sure everything functions as intended.
Debugging in a staging environment is easier, since its isolated from production. Security patches can also be tested before being deployed into production; having both environments allows software to be thoroughly evaluated before its release into use.
18. Adapting Software Development Standards
By customizing software development standards to suit specific project needs and evolving technologies, software standards provide a useful framework.
However, they should be tailored according to project needs, team composition and technological innovations that come up.
By tailoring development standards to each project, you can help guarantee its delivery on budget and on time. Aligning team strengths with specific project needs will facilitate efficient workflows while adapting software for new technologies keeps it current and relevant.
Finally, regular evaluations and updates of development standards ensure your team continues improving while producing top-quality software; one of the best software development workflow practices!
What Are The Best Practices For Your Project?
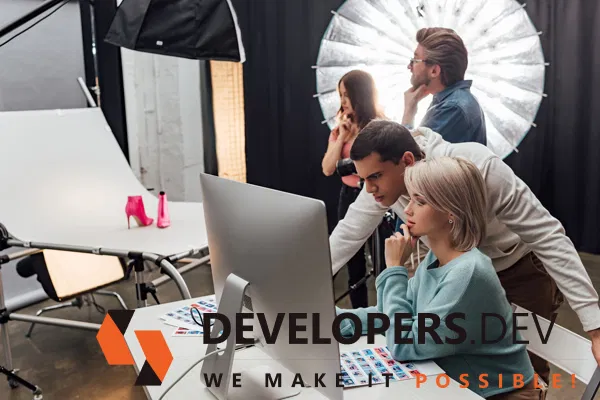
Adopting software development best practices will have a dramatic effect on your project, increasing its likelihood of success by making code efficient, scalable and maintainable - as well as decreasing security vulnerabilities or errors that arise during development - while simultaneously increasing team productivity and collaboration.
Agile methodologies such as Scrum can assist your teams ability to adapt quickly to changing requirements, while simultaneously improving communication and teamwork.
Automated tests and code reviews enable early error identification leading to less bugs being introduced during development; Git provides version control systems used by developers worldwide and manages code on behalf of the team.
By following best practices for secure software development, your team can produce more reliable and maintainable software projects.
This ensures everyone works effectively towards reaching the common goal of successfully delivering successful projects.
Want More Information About Our Services? Talk to Our Consultants!
Implementation Is The Key To Success
Software development services must implement these top 2023 software development best practices into their projects to ensure reliable and secure software products are created.
From planning and design through testing and deployment, these practices play an invaluable role.
Implementing these best practices will assist developers in improving collaboration, communication and productivity with team members and stakeholders while cutting costs, avoiding mistakes and meeting project deadlines more successfully.
Software development is an ever-evolving field and developers should always remain open-minded when learning new tools or techniques.
By regularly reviewing best practices for success, developers can stay current and ensure their projects run smoothly.